现有1500个二维空间的数据点,import time as time import numpy as np import matplotlib.pyplot as plt import mpl_toolkits.mplot3d.axes3d as p3 from sklearn.datasets import make_swiss_roll # Generate data (swiss roll dataset) n_samples = 1500 noise = 0.05 X, _ = make_swiss_roll(n_samples, noise=noise) fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.scatter(X[:, 0], X[:, 1], X[:, 2], cmap=plt.cm.Spectral),编写一个python程序不调用sklearn.manifold ,实现ISOMAP降维度
时间: 2024-02-25 12:59:40 浏览: 166
以下是一个简单的Python程序,用于实现ISOMAP算法对1500个二维空间数据点的降维处理:
```
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# Generate data
n_samples = 1500
noise = 0.05
X, _ = make_swiss_roll(n_samples, noise=noise)
# Define ISOMAP function
def isomap(data, k=7, d=2):
# Calculate pairwise distances between data points
dists = np.zeros((data.shape[0], data.shape[0]))
for i in range(data.shape[0]):
for j in range(i+1, data.shape[0]):
dists[i,j] = np.sqrt(np.sum((data[i] - data[j])**2))
dists[j,i] = dists[i,j]
# Construct neighborhood graph
knn_indices = np.argsort(dists)[:,:k+1]
knn_dists = np.array([dists[i, knn_indices[i]] for i in range(data.shape[0])])
knn_dists[:,0] = 0
knn_graph = np.zeros((data.shape[0], data.shape[0]))
for i in range(data.shape[0]):
knn_graph[i, knn_indices[i]] = knn_dists[i]
# Compute shortest path distances
inf = np.inf
dists[dists == 0] = inf
for k in range(data.shape[0]):
for i in range(data.shape[0]):
for j in range(data.shape[0]):
dists[i,j] = min(dists[i,j], dists[i,k] + dists[k,j])
# Compute low-dimensional embedding
dists[dists == inf] = 0
B = -0.5*(dists**2 - np.sum(dists**2, axis=0)/data.shape[0] - np.sum(dists**2, axis=1)/data.shape[0] + np.sum(dists**2)/data.shape[0]**2)
_, eigvecs = np.linalg.eigh(B)
embedding = eigvecs[:, :d]
return embedding
# Apply ISOMAP to data
embedding = isomap(X)
# Visualize results
fig = plt.figure()
ax = fig.add_subplot(111)
ax.scatter(embedding[:,0], embedding[:,1], cmap=plt.cm.Spectral)
plt.show()
```
该程序首先生成数据集,然后定义了一个名为isomap的函数来实现ISOMAP算法。该函数首先计算数据点之间的距离,然后构建邻域图,并使用Floyd-Warshall算法计算最短路径距离。最后,该函数将最短路径距离转换为低维嵌入,并返回嵌入结果。
该程序将ISOMAP算法应用于数据集,并使用2D散点图可视化了嵌入结果。
阅读全文
相关推荐
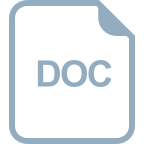
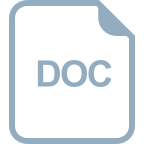
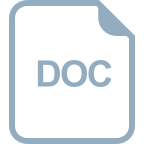















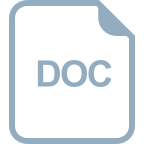