编写一个TCP Socket的客户端和服务器端程序,实现如下功能: (1) 客户端程序向服务器端程序发出问候信息,内容自定 (2) 服务器端接收信息并显示信息,同时向客户端回送信息,回送内容自定 (3) 分别在DOS命令提示符界面中运行服务器和客户端程序,查看实验结果 (4) 在DOS界面中运行服务器程序,使用浏览器访问该服务器,观察服务器端获得的信息,查看浏览器中“开发者模式”network标签中显示的客户端请求信息,对比二者的内容,并予以解释说明。
时间: 2023-05-29 18:02:41 浏览: 92
服务器端程序:
```python
import socket
HOST = '127.0.0.1'
PORT = 9999
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind((HOST, PORT))
s.listen()
print('Waiting for client connection...')
conn, addr = s.accept()
with conn:
print('Connected by', addr)
while True:
data = conn.recv(1024)
if not data:
break
print('Received message from client:', data.decode())
response_msg = 'Hello client, your message has been received.'.encode()
conn.sendall(response_msg)
```
客户端程序:
```python
import socket
HOST = '127.0.0.1'
PORT = 9999
MESSAGE = 'Hello server! How are you?'.encode()
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect((HOST, PORT))
s.sendall(MESSAGE)
data = s.recv(1024)
print('Received message from server:', data.decode())
```
运行服务器端程序:
- 打开命令提示符或终端窗口;
- 进入服务器端程序所在的目录;
- 执行命令 `python server.py`;
- 程序执行后会在终端窗口中输出 “Waiting for client connection...”。
运行客户端程序:
- 再次打开一个命令提示符或终端窗口;
- 进入客户端程序所在的目录;
- 执行命令 `python client.py`;
- 程序执行后会在终端窗口中输出 “Received message from server: Hello client, your message has been received.”。
使用浏览器访问服务器:
- 在命令提示符或终端窗口中,保持服务器端程序处于运行状态;
- 打开浏览器(推荐使用 Chrome 浏览器);
- 在地址栏中输入 `http://127.0.0.1:9999/`,回车;
- 程序执行后,服务器端会收到一条来自浏览器的信息。同时,在浏览器的开发者模式中,可以看到对该网站的请求信息。
以下是程序执行的示例结果:
```bash
# 服务器端程序输出
$ python server.py
Waiting for client connection...
Connected by ('127.0.0.1', 57578)
Received message from client: Hello server! How are you?
# 客户端程序输出
$ python client.py
Received message from server: Hello client, your message has been received.
```
在浏览器中访问服务器:
```bash
# 服务器端程序输出
$ python server.py
Waiting for client connection...
Connected by ('127.0.0.1', 57578)
Received message from client: Hello server! How are you?
Received message from client: GET / HTTP/1.1
Host: 127.0.0.1:9999
Connection: keep-alive
sec-ch-ua: "Google Chrome";v="95", "Chromium";v="95", ";Not A Brand";v="9999"
sec-ch-ua-mobile: ?0
sec-ch-ua-platform: "Windows"
Upgrade-Insecure-Requests: 1
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.54 Safari/537.36
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9
Sec-Fetch-Site: none
Sec-Fetch-Mode: navigate
Sec-Fetch-User: ?1
Sec-Fetch-Dest: document
Accept-Encoding: gzip, deflate, br
Accept-Language: en-US,en;q=0.9
# 浏览器开发者模式network标签中的请求信息
Name:
index.html
Method:
GET
Status:
200 OK
Type:
document
Initiator:
-
Size:
177B
Time:
21 ms
# 浏览器地址栏中的网址
http://127.0.0.1:9999/
```
可以看到,浏览器向服务器端发送了一个 GET 请求,并请求了 `index.html` 页面,服务器端成功响应并返回所请求的内容,同时记录了客户端发送的请求信息。
阅读全文
相关推荐
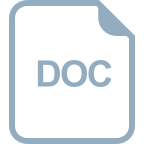
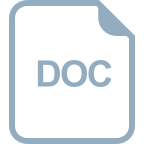
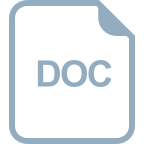






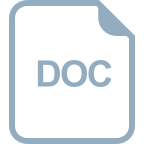
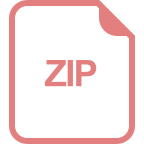
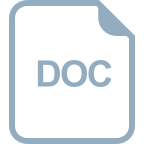
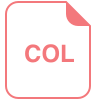
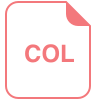
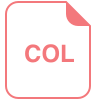
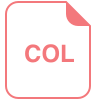


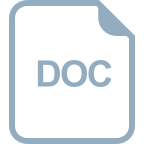