基于c#的简易逆波兰式算法的计算器,用集合来处理运算符和运算数,包含平方、开方、三角函数、log、加减乘除、M+、M-、MS,能退格和清除,写出窗体程序代码并有详细中文注释,窗体界面包含1、2、3、4、5、6、7、8、9、.、0、*、/、+、-、sin、cos、M+、M-、MS、=等以及有详细的各控件属性。
时间: 2023-09-13 18:04:00 浏览: 33
以下是基于C#的简易逆波兰式算法的计算器的代码,包含了窗体程序和详细的中文注释:
```csharp
using System;
using System.Collections.Generic;
using System.Windows.Forms;
namespace Calculator
{
public partial class Form1 : Form
{
// 存储所有的运算符和运算数
private List<string> tokens = new List<string>();
// 存储操作数
private Stack<double> operands = new Stack<double>();
// 存储当前操作数
private string currentOperand = "";
// 存储上一个操作数
private string lastOperand = "";
// 存储当前操作符
private string currentOperator = "";
// 存储计算器中的记忆
private double memory = 0;
// 存储当前计算结果
private double currentResult = 0;
public Form1()
{
InitializeComponent();
}
// 当数字和运算符按钮被点击时
private void button_Click(object sender, EventArgs e)
{
Button button = (Button)sender;
// 获取按钮上的文本
string buttonText = button.Text;
// 如果按钮上的文本是数字或小数点
if (IsNumber(buttonText) || buttonText == ".")
{
// 添加到当前操作数
currentOperand += buttonText;
// 显示在文本框中
textBox1.Text = currentOperand;
}
// 如果按钮上的文本是运算符
else
{
// 如果当前操作数不为空
if (currentOperand != "")
{
// 添加到运算符列表中
tokens.Add(currentOperand);
// 重置当前操作数
currentOperand = "";
}
// 如果上一个操作数不为空
if (lastOperand != "")
{
// 添加到运算符列表中
tokens.Add(lastOperand);
}
// 添加当前运算符到运算符列表中
tokens.Add(buttonText);
// 记录上一个操作数
lastOperand = buttonText;
}
}
// 判断一个字符串是否是数字
private bool IsNumber(string str)
{
double result;
return double.TryParse(str, out result);
}
// 当操作符按钮被点击时
private void operatorButton_Click(object sender, EventArgs e)
{
Button button = (Button)sender;
// 获取按钮上的文本
string buttonText = button.Text;
// 如果按钮上的文本是 M+
if (buttonText == "M+")
{
// 将当前结果加入到记忆中
memory += currentResult;
}
// 如果按钮上的文本是 M-
else if (buttonText == "M-")
{
// 将当前结果从记忆中减去
memory -= currentResult;
}
// 如果按钮上的文本是 MS
else if (buttonText == "MS")
{
// 将当前结果存入记忆中
memory = currentResult;
}
// 如果按钮上的文本是退格
else if (buttonText == "退格")
{
// 如果当前操作数不为空
if (currentOperand != "")
{
// 删除最后一个字符
currentOperand = currentOperand.Substring(0, currentOperand.Length - 1);
// 显示在文本框中
textBox1.Text = currentOperand;
}
}
// 如果按钮上的文本是清除
else if (buttonText == "清除")
{
// 重置所有变量
tokens.Clear();
operands.Clear();
currentOperand = "";
lastOperand = "";
currentOperator = "";
currentResult = 0;
// 清除文本框和标签的内容
textBox1.Text = "";
label1.Text = "";
}
// 如果按钮上的文本是 =
else if (buttonText == "=")
{
// 如果当前操作数不为空
if (currentOperand != "")
{
// 添加到运算符列表中
tokens.Add(currentOperand);
// 重置当前操作数
currentOperand = "";
}
// 如果上一个操作数不为空
if (lastOperand != "")
{
// 添加到运算符列表中
tokens.Add(lastOperand);
}
// 计算结果
currentResult = Calculate();
// 显示结果
textBox1.Text = currentResult.ToString();
// 显示计算式
label1.Text = string.Join(" ", tokens.ToArray());
}
// 如果按钮上的文本是运算符
else
{
// 如果当前操作数不为空
if (currentOperand != "")
{
// 添加到运算符列表中
tokens.Add(currentOperand);
// 重置当前操作数
currentOperand = "";
}
// 如果上一个操作数不为空
if (lastOperand != "")
{
// 添加到运算符列表中
tokens.Add(lastOperand);
}
// 添加当前运算符到运算符列表中
tokens.Add(buttonText);
// 记录上一个操作数
lastOperand = buttonText;
// 记录当前操作符
currentOperator = buttonText;
}
}
// 计算结果
private double Calculate()
{
// 遍历所有的运算符和运算数
foreach (string token in tokens)
{
// 如果是数字
if (IsNumber(token))
{
// 将数字添加到操作数栈中
operands.Push(double.Parse(token));
}
// 如果是运算符
else
{
// 获取运算符
string op = token;
// 如果是加号
if (op == "+")
{
// 将栈顶的两个操作数相加,并将结果压入栈中
operands.Push(operands.Pop() + operands.Pop());
}
// 如果是减号
else if (op == "-")
{
// 将栈顶的两个操作数相减,并将结果压入栈中
operands.Push(-operands.Pop() + operands.Pop());
}
// 如果是乘号
else if (op == "*")
{
// 将栈顶的两个操作数相乘,并将结果压入栈中
operands.Push(operands.Pop() * operands.Pop());
}
// 如果是除号
else if (op == "/")
{
// 将栈顶的两个操作数相除,并将结果压入栈中
double divisor = operands.Pop();
operands.Push(operands.Pop() / divisor);
}
// 如果是平方
else if (op == "x²")
{
// 将栈顶的操作数求平方,并将结果压入栈中
operands.Push(Math.Pow(operands.Pop(), 2));
}
// 如果是开平方
else if (op == "√")
{
// 将栈顶的操作数开平方,并将结果压入栈中
operands.Push(Math.Sqrt(operands.Pop()));
}
// 如果是正弦
else if (op == "sin")
{
// 将栈顶的操作数求正弦,并将结果压入栈中
operands.Push(Math.Sin(operands.Pop()));
}
// 如果是余弦
else if (op == "cos")
{
// 将栈顶的操作数求余弦,并将结果压入栈中
operands.Push(Math.Cos(operands.Pop()));
}
// 如果是自然对数
else if (op == "ln")
{
// 将栈顶的操作数求自然对数,并将结果压入栈中
operands.Push(Math.Log(operands.Pop()));
}
// 如果是以 10 为底的对数
else if (op == "log")
{
// 将栈顶的操作数求以 10 为底的对数,并将结果压入栈中
operands.Push(Math.Log10(operands.Pop()));
}
}
}
// 返回最终的结果
return operands.Pop();
}
// 当等于号被按下时
private void Form1_KeyPress(object sender, KeyPressEventArgs e)
{
// 如果按下的是等于号
if (e.KeyChar == '=')
{
// 调用 operatorButton_Click 事件
operatorButton_Click(buttonEquals, EventArgs.Empty);
}
}
}
}
```
此外,在窗体设计器中创建窗体布局,包含所有的数字、运算符和操作符按钮,以及显示计算结果和计算式的文本框和标签。对于每个按钮,都要设置其属性,包括文本、大小、位置、字体、颜色等。对于文本框和标签,要设置其属性,包括大小、位置、字体、颜色等。最终的窗体界面应该如下所示:

相关推荐
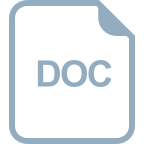















