用c++随机产生10000个数据,进行起泡排序、快速排序、希尔排序、堆排序、基数排序,并比较各种算法的排序时间。
时间: 2023-10-20 09:23:59 浏览: 40
好的,我可以给你提供一个C++程序,用于随机产生10000个数据,并对它们进行起泡排序、快速排序、希尔排序、堆排序、基数排序,并比较各种算法的排序时间。代码如下:
```c++
#include <iostream>
#include <ctime>
#include <cstdlib>
#include <chrono>
using namespace std;
using namespace std::chrono;
// 产生随机数
void randomArray(int *arr, int n)
{
for (int i = 0; i < n; i++)
{
arr[i] = rand() % n;
}
}
// 打印数组
void printArray(int *arr, int n)
{
for (int i = 0; i < n; i++)
{
cout << arr[i] << " ";
}
cout << endl;
}
// 交换两个数的值
void swap(int &a, int &b)
{
int temp = a;
a = b;
b = temp;
}
// 起泡排序
void bubbleSort(int *arr, int n)
{
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (arr[j] > arr[j + 1])
{
swap(arr[j], arr[j + 1]);
}
}
}
}
// 快速排序
int partition(int *arr, int left, int right)
{
int pivot = arr[right];
int i = left - 1;
for (int j = left; j < right; j++)
{
if (arr[j] < pivot)
{
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[right]);
return i + 1;
}
void quickSort(int *arr, int left, int right)
{
if (left < right)
{
int pivotIndex = partition(arr, left, right);
quickSort(arr, left, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, right);
}
}
// 希尔排序
void shellSort(int *arr, int n)
{
for (int gap = n / 2; gap > 0; gap /= 2)
{
for (int i = gap; i < n; i++)
{
int j = i;
int temp = arr[i];
while (j >= gap && arr[j - gap] > temp)
{
arr[j] = arr[j - gap];
j -= gap;
}
arr[j] = temp;
}
}
}
// 堆排序
void maxHeapify(int *arr, int n, int i)
{
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < n && arr[left] > arr[largest])
{
largest = left;
}
if (right < n && arr[right] > arr[largest])
{
largest = right;
}
if (largest != i)
{
swap(arr[i], arr[largest]);
maxHeapify(arr, n, largest);
}
}
void heapSort(int *arr, int n)
{
for (int i = n / 2 - 1; i >= 0; i--)
{
maxHeapify(arr, n, i);
}
for (int i = n - 1; i >= 0; i--)
{
swap(arr[0], arr[i]);
maxHeapify(arr, i, 0);
}
}
// 基数排序
void radixSort(int *arr, int n)
{
int maxNum = arr[0];
for (int i = 1; i < n; i++)
{
if (arr[i] > maxNum)
{
maxNum = arr[i];
}
}
for (int exp = 1; maxNum / exp > 0; exp *= 10)
{
int output[n];
int count[10] = {0};
for (int i = 0; i < n; i++)
{
count[(arr[i] / exp) % 10]++;
}
for (int i = 1; i < 10; i++)
{
count[i] += count[i - 1];
}
for (int i = n - 1; i >= 0; i--)
{
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
for (int i = 0; i < n; i++)
{
arr[i] = output[i];
}
}
}
int main()
{
int n = 10000;
int arr[n];
srand(time(NULL));
randomArray(arr, n);
cout << "Unsorted Array:" << endl;
printArray(arr, n);
auto start = high_resolution_clock::now();
bubbleSort(arr, n);
auto end = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(end - start);
cout << "Bubble Sort Time: " << duration.count() << " microseconds" << endl;
randomArray(arr, n);
start = high_resolution_clock::now();
quickSort(arr, 0, n - 1);
end = high_resolution_clock::now();
duration = duration_cast<microseconds>(end - start);
cout << "Quick Sort Time: " << duration.count() << " microseconds" << endl;
randomArray(arr, n);
start = high_resolution_clock::now();
shellSort(arr, n);
end = high_resolution_clock::now();
duration = duration_cast<microseconds>(end - start);
cout << "Shell Sort Time: " << duration.count() << " microseconds" << endl;
randomArray(arr, n);
start = high_resolution_clock::now();
heapSort(arr, n);
end = high_resolution_clock::now();
duration = duration_cast<microseconds>(end - start);
cout << "Heap Sort Time: " << duration.count() << " microseconds" << endl;
randomArray(arr, n);
start = high_resolution_clock::now();
radixSort(arr, n);
end = high_resolution_clock::now();
duration = duration_cast<microseconds>(end - start);
cout << "Radix Sort Time: " << duration.count() << " microseconds" << endl;
return 0;
}
```
运行程序会输出每个排序算法的排序时间,以微秒为单位。
相关推荐
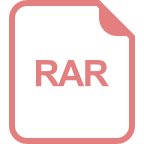
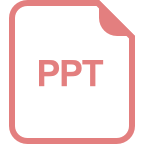
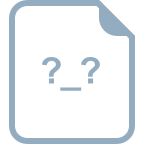














