easyx表达式求值文本框输入
时间: 2023-06-29 17:03:09 浏览: 124
你可以使用EasyX图形库提供的Edit类创建一个文本框,然后在用户输入数学表达式后,使用数学库计算表达式的值,并将结果输出到窗口中。
以下是一个示例代码:
```cpp
#include <graphics.h>
#include <conio.h>
#include <iostream>
#include <string>
#include <stack>
#include <cmath>
using namespace std;
double compute(string exp) {
stack<double> nums;
stack<char> ops;
for(int i = 0; i < exp.size(); i++) {
if(exp[i] == ' ')
continue;
if(exp[i] >= '0' && exp[i] <= '9') {
double num = 0;
while(i < exp.size() && ((exp[i] >= '0' && exp[i] <= '9') || exp[i] == '.')) {
if(exp[i] == '.') {
i++;
double frac = 1;
while(i < exp.size() && exp[i] >= '0' && exp[i] <= '9') {
frac /= 10;
num += (exp[i] - '0') * frac;
i++;
}
}
else {
num = num * 10 + (exp[i] - '0');
i++;
}
}
nums.push(num);
i--;
}
else if(exp[i] == '(')
ops.push(exp[i]);
else if(exp[i] == ')') {
while(ops.top() != '(') {
double num2 = nums.top();
nums.pop();
double num1 = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if(op == '+')
nums.push(num1 + num2);
else if(op == '-')
nums.push(num1 - num2);
else if(op == '*')
nums.push(num1 * num2);
else if(op == '/')
nums.push(num1 / num2);
}
ops.pop();
}
else {
while(!ops.empty() && ((exp[i] == '*' || exp[i] == '/') || (exp[i] == '+' || exp[i] == '-') && (ops.top() == '*' || ops.top() == '/'))) {
double num2 = nums.top();
nums.pop();
double num1 = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if(op == '+')
nums.push(num1 + num2);
else if(op == '-')
nums.push(num1 - num2);
else if(op == '*')
nums.push(num1 * num2);
else if(op == '/')
nums.push(num1 / num2);
}
ops.push(exp[i]);
}
}
while(!ops.empty()) {
double num2 = nums.top();
nums.pop();
double num1 = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
if(op == '+')
nums.push(num1 + num2);
else if(op == '-')
nums.push(num1 - num2);
else if(op == '*')
nums.push(num1 * num2);
else if(op == '/')
nums.push(num1 / num2);
}
return nums.top();
}
int main() {
initgraph(640, 480);
setbkcolor(WHITE);
cleardevice();
EditBox expBox(20, 20, 400, 30, true);
expBox.SetDefaultText("请输入数学表达式");
while(1) {
if(expBox.IsEnter()) {
string exp = expBox.GetText();
double result = compute(exp);
expBox.Clear();
expBox.SetDefaultText("请输入数学表达式");
char resultStr[50];
sprintf_s(resultStr, "%.2f", result);
outtextxy(20, 60, resultStr);
}
Sleep(10);
}
getch();
closegraph();
return 0;
}
```
这个示例中使用了一个compute函数,该函数可以计算一个字符串形式的数学表达式的值。在主函数中,创建了一个EditBox对象,用于接收用户输入的数学表达式,然后调用compute函数计算表达式的值,并将结果输出到窗口中。
阅读全文
相关推荐
















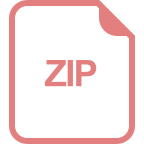