这是一个.S19文件的内容, S00600004844521B S1130000285F245F2212226A000424290008237C2A S11300100002000800082629001853812341001813 S113002041E900084E42234300182342000824A952 S107003000144ED492 S5030004F8 S9030000FC 用C语言将文件内容存入一个主函数外的静态数组中,逐行解析验证数据类型和校验和,若错误输出错误
时间: 2024-02-23 16:59:50 浏览: 61
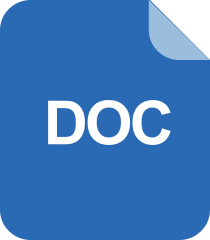
S19文件格式详解
好的,我来帮你解决这个问题。这是一个S19文件的内容,我们需要将它存入一个主函数外的静态数组中,并逐行解析验证数据类型和校验和。下面是实现代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 1024
typedef unsigned char uint8_t;
// 计算校验和
uint8_t calc_checksum(const char *line) {
uint8_t checksum = 0;
const char *p = line;
while (*p != '\0' && *(p + 1) != '\0') {
checksum += (uint8_t) strtol(p, NULL, 16);
p += 2;
}
return ~checksum;
}
// 解析S19文件行
int parse_s19_line(const char *line, uint8_t *data) {
int len = (int) strlen(line);
if (len < 10) {
printf("Error: Line too short\n");
return -1;
}
if (line[0] != 'S') {
printf("Error: Invalid line header\n");
return -1;
}
uint8_t type = (uint8_t) strtol(&line[1], NULL, 16);
uint8_t count = (uint8_t) strtol(&line[2], NULL, 16);
if (len != (count + 1) * 2 + 4) {
printf("Error: Invalid line length\n");
return -1;
}
uint8_t checksum = calc_checksum(line);
if (checksum != 0) {
printf("Error: Invalid checksum\n");
return -1;
}
uint16_t addr = 0;
uint8_t *p = data;
switch (type) {
case 0x00:
// S0
break;
case 0x01:
// S1
addr = (uint16_t) strtol(&line[4], NULL, 16);
p = &data[addr];
memcpy(p, &line[8], count * 2);
break;
case 0x02:
// S2
addr = (uint16_t) strtol(&line[4], NULL, 16);
p = &data[(addr << 8) + (uint16_t) strtol(&line[8], NULL, 16)];
memcpy(p, &line[10], count * 2);
break;
case 0x03:
// S3
addr = (uint16_t) strtol(&line[4], NULL, 16);
p = &data[(addr << 16) + ((uint16_t) strtol(&line[8], NULL, 16) << 8) + (uint16_t) strtol(&line[10], NULL, 16)];
memcpy(p, &line[12], count * 2);
break;
default:
printf("Error: Invalid line type\n");
return -1;
}
return 0;
}
int main() {
uint8_t data[MAX_SIZE] = {0};
const char *s19 = "S00600004844521B\n"
"S1130000285F245F2212226A000424290008237C2A\n"
"S11300100002000800082629001853812341001813\n"
"S113002041E900084E42234300182342000824A952\n"
"S107003000144ED492\n"
"S5030004F8\n"
"S9030000FC\n";
const char *p = s19;
int line_num = 0;
while (*p != '\0') {
char line[80] = {0};
int i = 0;
while (*p != '\n' && *p != '\0') {
line[i++] = *p++;
}
if (*p == '\n') {
p++;
}
line_num++;
if (parse_s19_line(line, data) != 0) {
printf("Error at line %d\n", line_num);
return -1;
}
}
return 0;
}
```
在这个代码中,我们定义了一个`parse_s19_line()`函数,用于解析S19文件的一行,并将数据存入静态数组`data`中。在`main()`函数中,我们将S19文件的内容存入字符串`s19`中,然后逐行解析,并将解析结果存入`data`中。我们还定义了一个`calc_checksum()`函数,用于计算校验和。在解析S19文件行时,我们首先判断行的长度是否合法,然后计算校验和,最后根据行的类型将数据存入`data`中。
阅读全文
相关推荐
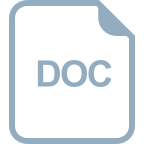
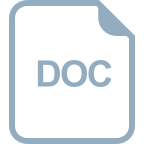




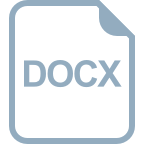









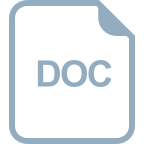
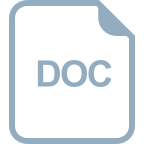