Spring Boot文件上传与下载的实现
时间: 2023-05-29 07:06:10 浏览: 117
1. 文件上传
Spring Boot提供了MultipartFile接口来处理文件上传。首先需要在HTML页面中添加一个表单来上传文件:
```html
<form method="POST" enctype="multipart/form-data" action="/upload">
<input type="file" name="file"/>
<button type="submit">上传</button>
</form>
```
然后在后端使用@RequestParam注解来获取上传的文件,如下所示:
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
try {
// 获取文件名
String fileName = file.getOriginalFilename();
// 获取文件内容
byte[] bytes = file.getBytes();
// 处理文件内容
// ...
return "上传成功";
} catch (IOException e) {
e.printStackTrace();
return "上传失败";
}
}
```
2. 文件下载
Spring Boot提供了Resource接口来处理文件下载。首先需要在HTML页面中添加一个链接来触发文件下载:
```html
<a href="/download?fileName=example.txt">下载文件</a>
```
然后在后端使用@PathVariable注解来获取文件名,如下所示:
```java
@GetMapping("/download/{fileName:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName) {
try {
// 获取文件
Resource resource = new FileSystemResource("path/to/file/" + fileName);
// 设置响应类型
String contentType = Files.probeContentType(resource.getFile().toPath());
// 构建响应对象
return ResponseEntity.ok()
.contentType(MediaType.parseMediaType(contentType))
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
}
```
其中,使用FileSystemResource来获取文件资源,使用Files.probeContentType来获取文件类型,构建响应对象时设置了响应类型、下载文件名等信息。最后将文件内容封装为ResponseEntity对象,返回给浏览器实现文件下载。
阅读全文
相关推荐
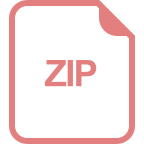
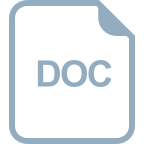
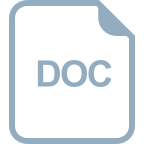
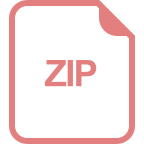
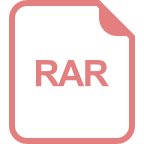
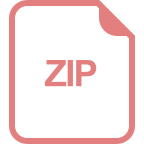
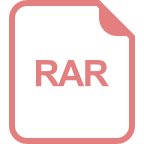
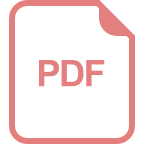
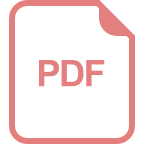
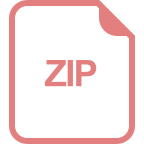
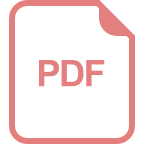
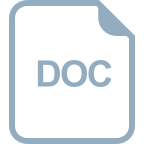
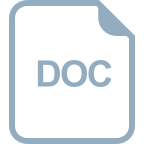