import pandas as pd def run_length_encoding(values): """使用游程编码计算值的游程长度""" rle_values = [] count = 1 for i in range(1, len(values)): if values[i] != values[i-1]: rle_values.append(count) count = 1 else: count += 1 rle_values.append(count) return rle_values def run_length_decoding(rle_values): """使用游程解码计算值的游程""" values = [] for i in range(len(rle_values)): values += [i % 2] * rle_values[i] return values def find_drought_events(rle_values, threshold): """使用游程理论找到干旱事件""" events = [] start = 0 for i in range(len(rle_values)): if rle_values[i] >= threshold and start == 0: start = sum(rle_values[:i]) elif rle_values[i] < threshold and start > 0: end = sum(rle_values[:i]) events.append((start, end)) start = 0 if start > 0: events.append((start, sum(rle_values))) return events # 从文件中读取数据 data = pd.read_csv('drought.csv') state_data = data[data['State'] == 'California'] state_data['Month'] = pd.to_datetime(state_data['Week'], format='%Y-%m-%d').dt.to_period('M') # 计算每个月的干旱指数 monthly_data = state_data.groupby('Month')['Value'].mean() # 计算游程长度 rle_values = run_length_encoding([1 if v < 0 else 0 for v in monthly_data.values]) # 计算干旱事件的开始和结束时间 drought_events = find_drought_events(rle_values, 3) # 输出结果 for event in drought_events: start_month = monthly_data.index[event[0]].strftime('%Y-%m') end_month = monthly_data.index[event[1]-1].strftime('%Y-%m') print(f"Drought event from {start_month} to {end_month}")解释代码
时间: 2023-06-14 15:06:13 浏览: 136
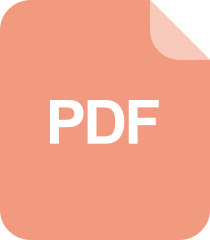
pandas read_excel()和to_excel()函数解析
这段代码主要是针对一个干旱指数数据集进行游程编码和游程解码,并使用游程理论找到干旱事件。
首先,代码从文件中读取数据,选择加利福尼亚州的数据,并将周数据转换为月数据。然后,计算每个月的干旱指数,将其存储在变量`monthly_data`中。
接下来,使用函数`run_length_encoding`对`monthly_data`进行游程编码,将每个游程的长度存储在变量`rle_values`中。
然后,使用函数`find_drought_events`基于游程理论找到干旱事件。该函数首先将游程长度序列作为输入,以及阈值参数。如果游程长度超过阈值并且当前没有干旱事件,则将该点标记为事件的开始。如果游程长度小于阈值并且当前有干旱事件,则将该点标记为事件的结束。最终,产生的干旱事件起始点和终止点被存储在变量`drought_events`中。
最后,代码遍历所有干旱事件,并输出它们的开始和结束时间。
需要注意的是,这段代码只是一个示例,如果想要应用到其他数据集中,需要对代码进行相应的修改。
阅读全文
相关推荐
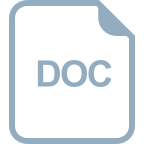
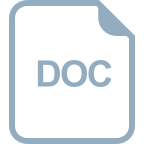
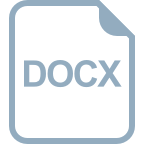
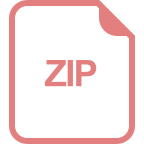
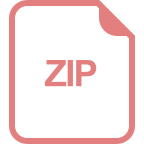
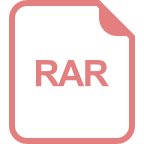
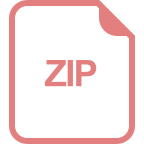
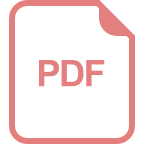
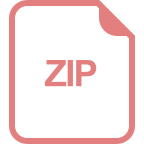
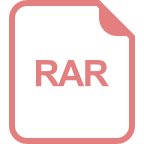
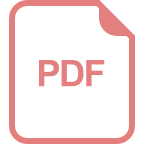
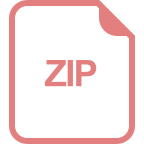
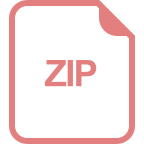
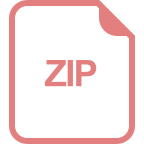
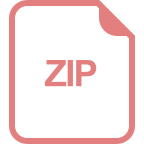
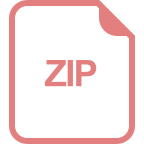
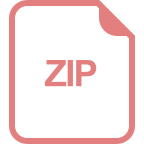
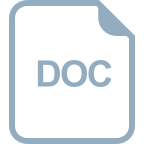