卡尔曼滤波sfunction
时间: 2023-10-14 22:05:35 浏览: 102
卡尔曼滤波是一种用于估计动态系统状态的数学方法,常用于信号处理和控制系统中。S-Function是Simulink中的一种功能块,用于自定义模型和算法。
将卡尔曼滤波应用于S-Function的步骤如下:
1. 在Simulink模型中创建一个S-Function块。
2. 在S-Function的回调函数中定义卡尔曼滤波算法。
3. 定义输入和输出端口以及其他所需的参数。
4. 在模型中使用S-Function来处理信号,实现卡尔曼滤波。
具体实现卡尔曼滤波的S-Function代码会根据具体应用和系统模型而有所不同。你可以参考Simulink的文档和示例来了解更多关于S-Function和卡尔曼滤波的相关信息。
相关问题
生成一段基于ROS的扩展卡尔曼滤波算法代码,要求订阅小车的里程计数据并通过扩展卡尔曼滤波算法来估算出下一时刻小车的x轴和y轴坐标
以下是一个基于ROS的扩展卡尔曼滤波算法示例代码,用于订阅小车的里程计数据并通过扩展卡尔曼滤波算法来估算下一时刻小车的x轴和y轴坐标。
```cpp
#include <ros/ros.h>
#include <nav_msgs/Odometry.h>
#include <geometry_msgs/PointStamped.h>
#include <geometry_msgs/PoseWithCovarianceStamped.h>
#include <tf/transform_datatypes.h>
#include <Eigen/Core>
#include <Eigen/LU>
// Define the state vector and covariance matrix
Eigen::VectorXd x(3);
Eigen::MatrixXd P(3, 3);
// Define the measurement noise covariance matrix R
Eigen::MatrixXd R(2, 2);
// Define the process noise covariance matrix Q
Eigen::MatrixXd Q(3, 3);
// Define the state transition matrix F and measurement matrix H
Eigen::MatrixXd F(3, 3);
Eigen::MatrixXd H(2, 3);
// Initialize the flag for the first time receiving odom data
bool odom_init = false;
// Initialize the last time stamp of the odom data
ros::Time last_odom_time;
// Callback function for the odom data
void odomCallback(const nav_msgs::Odometry::ConstPtr& odom_msg)
{
// Initialize the state vector x and covariance matrix P using the first odom data
if (!odom_init)
{
x << odom_msg->pose.pose.position.x,
odom_msg->pose.pose.position.y,
tf::getYaw(odom_msg->pose.pose.orientation);
P.setIdentity();
odom_init = true;
}
// Compute the time interval between the current and last odom data
double delta_t = (odom_msg->header.stamp - last_odom_time).toSec();
last_odom_time = odom_msg->header.stamp;
// Update the state transition matrix F and process noise covariance matrix Q
F << 1, 0, -sin(x(2))*odom_msg->twist.twist.linear.x*delta_t,
0, 1, cos(x(2))*odom_msg->twist.twist.linear.x*delta_t,
0, 0, 1;
Q << pow(delta_t, 4)/4, 0, pow(delta_t, 3)/2,
0, pow(delta_t, 4)/4, pow(delta_t, 3)/2,
pow(delta_t, 3)/2, pow(delta_t, 3)/2, pow(delta_t, 2);
// Predict the state vector x and covariance matrix P
Eigen::VectorXd x_pred = F*x;
Eigen::MatrixXd P_pred = F*P*F.transpose() + Q;
// Update the measurement matrix H and measurement noise covariance matrix R
H << 1, 0, 0,
0, 1, 0;
R << 0.1, 0,
0, 0.1;
// Convert the odom data to a PointStamped message for publishing
geometry_msgs::PointStamped point_msg;
point_msg.header.stamp = odom_msg->header.stamp;
point_msg.header.frame_id = odom_msg->header.frame_id;
point_msg.point.x = x_pred(0);
point_msg.point.y = x_pred(1);
point_msg.point.z = 0;
// Convert the state vector x and covariance matrix P to a PoseWithCovarianceStamped message for publishing
geometry_msgs::PoseWithCovarianceStamped pose_msg;
pose_msg.header.stamp = odom_msg->header.stamp;
pose_msg.header.frame_id = odom_msg->header.frame_id;
pose_msg.pose.pose.position.x = x_pred(0);
pose_msg.pose.pose.position.y = x_pred(1);
pose_msg.pose.pose.position.z = 0;
pose_msg.pose.pose.orientation = tf::createQuaternionMsgFromYaw(x_pred(2));
pose_msg.pose.covariance[0] = P_pred(0, 0);
pose_msg.pose.covariance[1] = P_pred(0, 1);
pose_msg.pose.covariance[5] = P_pred(1, 1);
pose_msg.pose.covariance[6] = P_pred(0, 2);
pose_msg.pose.covariance[7] = P_pred(1, 2);
pose_msg.pose.covariance[11] = P_pred(2, 2);
// Publish the PointStamped and PoseWithCovarianceStamped messages
ros::NodeHandle nh;
ros::Publisher point_pub = nh.advertise<geometry_msgs::PointStamped>("pose_estimate", 1);
ros::Publisher pose_pub = nh.advertise<geometry_msgs::PoseWithCovarianceStamped>("pose_estimate_cov", 1);
point_pub.publish(point_msg);
pose_pub.publish(pose_msg);
// Update the state vector x and covariance matrix P using the measurement data
Eigen::VectorXd z(2);
z << odom_msg->pose.pose.position.x,
odom_msg->pose.pose.position.y;
Eigen::VectorXd y = z - H*x_pred;
Eigen::MatrixXd S = H*P_pred*H.transpose() + R;
Eigen::MatrixXd K = P_pred*H.transpose()*S.inverse();
x = x_pred + K*y;
P = (Eigen::MatrixXd::Identity(3, 3) - K*H)*P_pred;
}
int main(int argc, char** argv)
{
ros::init(argc, argv, "ekf_node");
ros::NodeHandle nh;
// Subscribe to the odom data
ros::Subscriber odom_sub = nh.subscribe("odom", 1, odomCallback);
ros::spin();
return 0;
}
```
在这个示例代码中,我们使用了Eigen库来实现矩阵运算。首先,我们定义了状态向量x、协方差矩阵P、测量噪声协方差矩阵R和过程噪声协方差矩阵Q。然后,在odomCallback回调函数中,我们根据接收到的里程计数据更新状态向量x和协方差矩阵P。接着,我们根据更新后的状态向量x和协方差矩阵P,用扩展卡尔曼滤波算法预测下一时刻小车的x轴和y轴坐标,并将预测结果发布为一个PointStamped和PoseWithCovarianceStamped消息。最后,我们使用接收到的里程计数据来更新状态向量x和协方差矩阵P。
这个示例代码中假设小车只能直线行驶,因此状态向量x只包含了小车的x轴和y轴坐标以及方向角度。如果需要考虑小车的转向和加速度等因素,需要根据实际情况修改状态向量、协方差矩阵、过程噪声协方差矩阵和状态转移矩阵。
simulink 卡尔曼滤波
卡尔曼滤波是一种常用于估计系统状态的最优滤波方法,而Simulink是一款功能强大的系统建模和仿真工具。在Simulink中,可以借助Stateflow或者自定义的S-Function来实现卡尔曼滤波器。
首先,需要在Simulink中建立系统模型,并将模型的输入和输出与卡尔曼滤波器连接起来。可以使用输入信号作为测量值,经过卡尔曼滤波器后得到估计的状态值作为模型输出。可以使用Simulink中的Block来实现滤波器的输入和输出连接。
然后,需要构建卡尔曼滤波器模型。可以使用Simulink中的Transfer Fcn Block或者State-Space Block来表示卡尔曼滤波器的状态空间模型。也可以使用自定义的S-Function来实现更复杂的滤波器模型。
最后,需要设置卡尔曼滤波器的参数,如系统的初始状态、测量噪声、过程噪声等。这些参数可以通过Simulink中的Constant Block或者From Workspace Block来设定。
综上所述,Simulink可以通过连接模型的输入和输出,并使用合适的滤波器模型和参数来实现卡尔曼滤波。具体实现方式可以根据实际需求和系统模型的复杂度进行选择和调整。
阅读全文
相关推荐
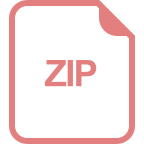
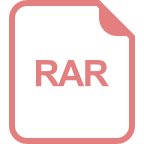
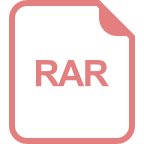
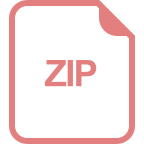
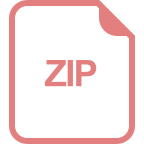
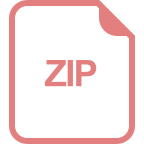
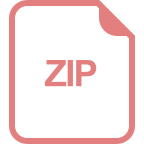
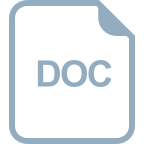
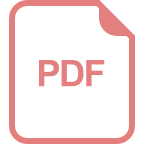
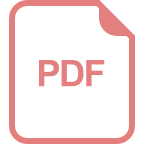
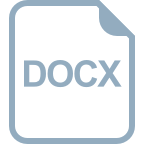
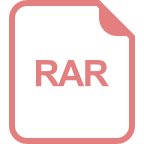
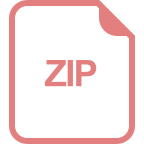
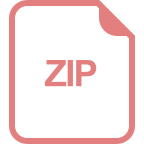
