ChannelInboundHandlerAdapter和SimpleChannelInboundHandler有什么区别
时间: 2023-12-28 19:03:30 浏览: 28
ChannelInboundHandlerAdapter和SimpleChannelInboundHandler都是Netty中的ChannelInboundHandler接口的实现类。
区别在于:
1. ChannelInboundHandlerAdapter是一个抽象类,而SimpleChannelInboundHandler是一个泛型类。
2. ChannelInboundHandlerAdapter中的方法都是空实现,需要用户自己实现需要的方法。而SimpleChannelInboundHandler中的channelRead0()方法已经被实现,用户只需要重写这个方法即可。
3. SimpleChannelInboundHandler比ChannelInboundHandlerAdapter更加方便,因为它可以自动释放ByteBuf对象,避免内存泄漏问题。而ChannelInboundHandlerAdapter需要手动释放ByteBuf对象。
因此,如果用户需要自己实现所有的方法,或者需要手动释放ByteBuf对象,可以使用ChannelInboundHandlerAdapter。如果用户只需要重写channelRead0()方法,并希望自动释放ByteBuf对象,可以使用SimpleChannelInboundHandler。
相关问题
new SimpleChannelInboundHandler
SimpleChannelInboundHandler是一个抽象类,继承自ChannelInboundHandlerAdapter。\[1\]它是Netty框架中的一个关键组件,用于处理入站消息。SimpleChannelInboundHandler在ChannelInboundHandlerAdapter的基础上新增了或重写了一些方法,其中最重要的是channelRead0方法。这个方法用于处理接收到的消息,并且只会处理特定类型的消息,而不需要手动释放资源。\[2\]
在Netty中,SimpleChannelInboundHandler通常用于客户端,而ChannelInboundHandlerAdapter通常用于服务端。这是因为SimpleChannelInboundHandler在处理完消息后会自动释放资源,而ChannelInboundHandlerAdapter需要手动释放资源。所以在Echo示例中,服务端使用ChannelInboundHandlerAdapter,而客户端使用SimpleChannelInboundHandler。\[3\]
总结来说,SimpleChannelInboundHandler是Netty框架中用于处理入站消息的抽象类,它继承自ChannelInboundHandlerAdapter并新增了一些方法,其中最重要的是channelRead0方法。在实际应用中,SimpleChannelInboundHandler通常用于客户端,而ChannelInboundHandlerAdapter通常用于服务端。
#### 引用[.reference_title]
- *1* *2* *3* [深入理解SimpleChannelInboundHandler](https://blog.csdn.net/u010739551/article/details/89496731)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
SimpleChannelInboundHandler如何使用?
SimpleChannelInboundHandler是Netty中的一个处理器,用于处理入站消息。它是ChannelInboundHandlerAdapter的子类,与其不同的是,SimpleChannelInboundHandler可以自动释放接收到的消息,在处理完消息后不需要手动释放。
使用SimpleChannelInboundHandler需要重写它的channelRead0方法,该方法会在接收到入站消息时被调用。在该方法中,可以处理接收到的消息并发送响应。
以下是一个使用SimpleChannelInboundHandler的示例:
```java
public class MyHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
// 处理接收到的消息
String response = "Hello, " + msg;
// 发送响应
ctx.writeAndFlush(response);
}
}
```
在上面的示例中,MyHandler继承了SimpleChannelInboundHandler,并重写了它的channelRead0方法。该方法会接收到一个ChannelHandlerContext对象和一个String类型的消息,其中ChannelHandlerContext对象包含了与客户端通信的上下文信息,可以用它来发送响应。在方法中,将接收到的消息拼接成响应,并使用ctx.writeAndFlush方法发送响应。
使用SimpleChannelInboundHandler需要将其添加到ChannelPipeline中,可以使用如下代码:
```java
public class MyServer {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new MyHandler());
}
});
ChannelFuture future = bootstrap.bind(8888).sync();
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
在上面的示例中,将MyHandler添加到了ChannelInitializer中,然后将ChannelInitializer添加到了ServerBootstrap中。这样,在接收到客户端的连接时,MyHandler就会被添加到该连接的ChannelPipeline中,用于处理接收到的消息。
相关推荐
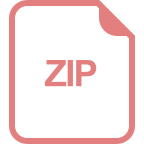














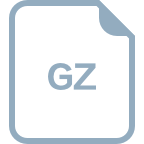