需要从一个水果工厂获得水果,其中“A”表示苹果,“P”代表菠萝,“O”代表橘子。请采用简单工厂设计模式进行设计,画出类图并写出源码。
时间: 2024-10-21 11:02:16 浏览: 8
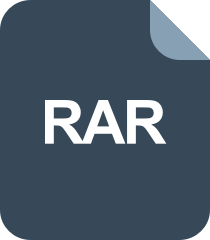
水果分类系统的matlab仿真区分出苹果、香蕉、菠萝等常见水果+含代码操作演示视频

简单工厂设计模式是一种创建型设计模式,它提供了一个创建对象的最佳方式,而不必暴露其内部创建逻辑。在这种情况下,我们可以创建一个 FruitFactory 类,该类包含对应每个水果类型的静态方法,然后返回对应的 Fruit 对象。
首先,我们定义一个基础的 Fruit 接口:
```java
// 水果接口
public interface Fruit {
String getName();
}
```
接着,为每种水果创建具体的实现类:
```java
// 苹果类
public class Apple implements Fruit {
private static final String NAME = "Apple";
@Override
public String getName() {
return NAME;
}
}
// 菠萝类
public class Pineapple implements Fruit {
private static final String NAME = "Pineapple";
@Override
public String getName() {
return NAME;
}
}
// 橘子类
public class Orange implements Fruit {
private static final String NAME = "Orange";
@Override
public String getName() {
return NAME;
}
}
```
现在,我们创建 Factory 类:
```java
// 水果工厂类
public class FruitFactory {
// 创建水果对象的方法
public static Fruit createFruit(String type) {
if (type.equalsIgnoreCase("A")) {
return new Apple();
} else if (type.equalsIgnoreCase("P")) {
return new Pineapple();
} else if (type.equalsIgnoreCase("O")) {
return new Orange();
} else {
throw new IllegalArgumentException("Invalid fruit type");
}
}
}
```
类图大致如下:
```
+--+
| -getName(): String|
+----------------+
|
v
+---------+ +---------+
| Apple | | Orange |
+---------+ +---------+
| |
V V
+-----------------+ +-----------------+
| FruitFactory.java| | FruitFactory.java|
+-----------------+ +-----------------+
```
阅读全文
相关推荐
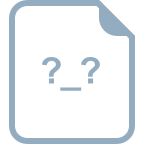
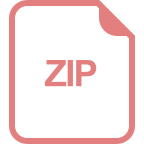

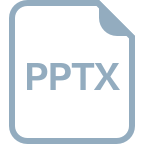
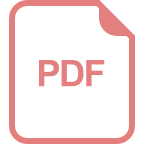
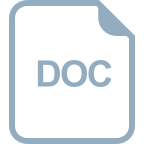
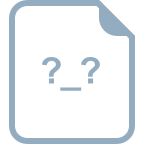
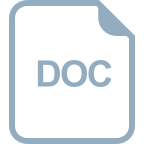
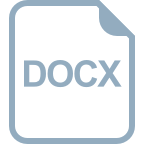
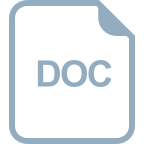
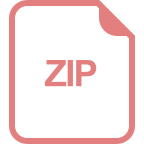
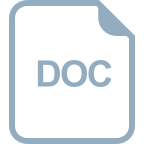
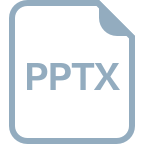
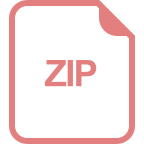
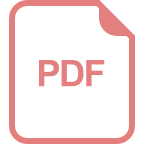
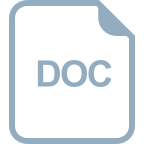
