1.用C语言不用C++语言,编写一个程序sqstack.c,实现顺序栈的各种基本运算(假设栈中元素类型ElemType为char)
时间: 2024-06-13 11:04:48 浏览: 129
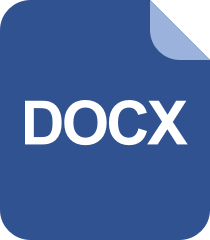
数据结构c语言版.docx
顺序栈是一种基于数组实现的栈结构,它的特点是后进先出,即最后一个入栈的元素最先出栈。下面是一个用C语言实现顺序栈的程序,其中包括了初始化栈、判断栈是否为空、入栈、出栈、取栈顶元素和释放栈等基本运算。
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100 // 定义栈的最大容量
typedef char ElemType; // 定义栈中元素类型为char
typedef struct {
ElemType data[MAXSIZE]; // 存储栈中元素的数组
int top; // 栈顶指针,指向栈顶元素的下标
} SqStack; // 定义顺序栈类型
// 初始化栈
void InitStack(SqStack *S) {
S->top = -1; // 将栈顶指针初始化为-1,表示栈为空
}
// 判断栈是否为空
int StackEmpty(SqStack S) {
if (S.top == -1) {
return 1; // 栈为空,返回1
} else {
return 0; // 栈不为空,返回0
}
}
// 入栈
int Push(SqStack *S, ElemType e) {
if (S->top == MAXSIZE - 1) {
return 0; // 栈满,入栈失败,返回0
} else {
S->top++; // 栈顶指针加1
S->data[S->top] = e; // 将元素e压入栈顶
return 1; // 入栈成功,返回1
}
}
// 出栈
int Pop(SqStack *S, ElemType *e) {
if (S->top == -1) {
return 0; // 栈空,出栈失败,返回0
} else {
*e = S->data[S->top]; // 将栈顶元素赋值给e
S->top--; // 栈顶指针减1
return 1; // 出栈成功,返回1
}
}
// 取栈顶元素
int GetTop(SqStack S, ElemType *e) {
if (S.top == -1) {
return 0; // 栈空,取栈顶元素失败,返回0
} else {
*e = S.data[S.top]; // 将栈顶元素赋值给e
return 1; // 取栈顶元素成功,返回1
}
}
// 释放栈
void DestroyStack(SqStack *S) {
S->top = -1; // 将栈顶指针初始化为-1,表示栈为空
}
int main() {
SqStack S;
ElemType e;
InitStack(&S); // 初始化栈
if (StackEmpty(S)) {
printf("栈为空\n"); // 判断栈是否为空
}
Push(&S, 'a'); // 入栈
Push(&S, 'b');
Push(&S, 'c');
Push(&S, 'd');
Push(&S, 'e');
if (!StackEmpty(S)) {
printf("栈不为空\n"); // 判断栈是否为空
}
printf("出栈序列:");
while (Pop(&S, &e)) {
printf("%c ", e); // 输出出栈序列
}
printf("\n");
if (StackEmpty(S)) {
printf("栈为空\n"); // 判断栈是否为空
}
DestroyStack(&S); // 释放栈
return 0;
}
```
阅读全文
相关推荐
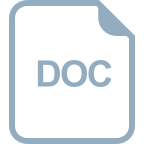
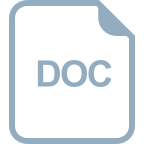















