1.用c语言实现顺序栈的各种基本运算的算法 编写一个程序sqstack.cpp,实现顺序栈的各种基本运算(假设栈中元素类型ElemType为char)
时间: 2024-06-13 08:04:52 浏览: 178
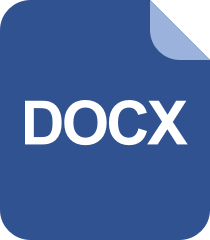
数据结构c语言版.docx
顺序栈是一种基于数组实现的栈结构,它具有后进先出(LIFO)的特点。下面是实现顺序栈的各种基本运算的算法:
1. 初始化栈:定义一个数组作为栈的存储空间,再定义一个变量top表示栈顶指针,初始值为-1。
2. 判断栈是否为空:当top等于-1时,栈为空。
3. 判断栈是否已满:当top等于数组长度减1时,栈已满。
4. 入栈操作:将元素插入到栈顶,即将top加1,再将元素赋值给数组中top所指向的位置。
5. 出栈操作:将栈顶元素弹出,即将top减1,再返回数组中top所指向的元素。
6. 取栈顶元素:返回数组中top所指向的元素。
下面是sqstack.cpp的实现代码:
```
#include <iostream>
using namespace std;
#define MAXSIZE 100 // 定义栈的最大长度
typedef char ElemType; // 定义栈中元素类型为char
typedef struct {
ElemType data[MAXSIZE]; // 存储栈中元素的数组
int top; // 栈顶指针
} SqStack;
// 初始化栈
void InitStack(SqStack &S) {
S.top = -1;
}
// 判断栈是否为空
bool StackEmpty(SqStack S) {
return S.top == -1;
}
// 判断栈是否已满
bool StackFull(SqStack S) {
return S.top == MAXSIZE - 1;
}
// 入栈操作
bool Push(SqStack &S, ElemType x) {
if (StackFull(S)) {
return false;
}
S.top++;
S.data[S.top] = x;
return true;
}
// 出栈操作
bool Pop(SqStack &S, ElemType &x) {
if (StackEmpty(S)) {
return false;
}
x = S.data[S.top];
S.top--;
return true;
}
// 取栈顶元素
bool GetTop(SqStack S, ElemType &x) {
if (StackEmpty(S)) {
return false;
}
x = S.data[S.top];
return true;
}
int main() {
SqStack S;
InitStack(S);
Push(S, 'a');
Push(S, 'b');
Push(S, 'c');
ElemType x;
Pop(S, x);
cout << x << endl; // 输出c
GetTop(S, x);
cout << x << endl; // 输出b
return 0;
}
```
阅读全文
相关推荐
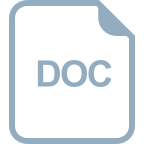
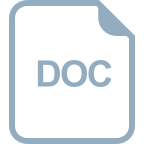















