Python编写一个比较两个文件内容是否相同的程序,若相同,显示“compare ok”,否则,显示“not equal”。
时间: 2024-10-10 19:10:39 浏览: 25
在Python中,你可以使用内置的`open()`函数读取文件的内容,然后将它们存储在字符串变量中进行比较。下面是一个简单的程序示例,它会逐行对比两个文本文件:
```python
def compare_files(file1_path, file2_path):
with open(file1_path, 'r') as file1, open(file2_path, 'r') as file2:
content1 = file1.readlines()
content2 = file2.readlines()
if content1 == content2:
print("compare ok")
else:
print("not equal")
# 使用方法
compare_files('file1.txt', 'file2.txt')
```
这个程序首先打开两个文件并读取所有内容到列表中。然后通过比较这两个列表是否相等来判断两文件内容是否一致。如果相等,则打印"compare ok",否则打印"not equal"。
请注意,这个程序假设两个文件大小相同并且都是文本文件。如果文件大小不同或包含二进制数据,需要对处理方式进行调整。
相关问题
python编写一个比较两个文件内容是否相同的程序,若相同,显示“compare ok”,否则,显示“not equal”
可以使用以下Python代码来实现:
```python
file1 = input("请输入要比较的第一个文件名:")
file2 = input("请输入要比较的第二个文件名:")
with open(file1, 'r') as f1, open(file2, 'r') as f2:
if f1.read() == f2.read():
print("compare ok")
else:
print("not equal")
```
这段代码中,首先通过 `input()` 函数获取要比较的两个文件名,然后使用 `with open()` 语句打开这两个文件,并分别将它们的内容读取出来进行比较。如果两个文件的内容相同,则输出 "compare ok",否则输出 "not equal"。
编写一个比较两个文件内容是否相同的程序,若相同,显示“compare ok”,否则,显示“not equal”python
在Python中,你可以使用内置模块`difflib`来比较两个文件的内容。下面是一个简单的程序示例:
```python
import difflib
def compare_files(file1_path, file2_path):
with open(file1_path, 'r') as file1, open(file2_path, 'r') as file2:
file1_content = file1.read().splitlines()
file2_content = file2.read().splitlines()
differ = difflib.Differ()
diff = list(differ.compare(file1_content, file2_content))
if not diff:
print("compare ok")
else:
print("not equal")
# 替换为实际文件路径
file1_path = "path_to_file1.txt"
file2_path = "path_to_file2.txt"
compare_files(file1_path, file2_path)
阅读全文
相关推荐
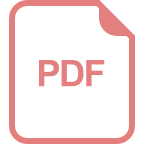
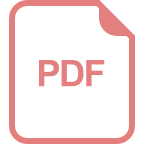
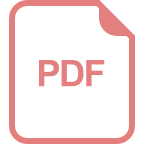



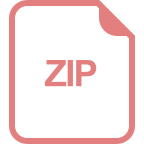
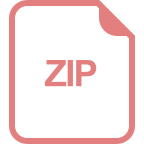
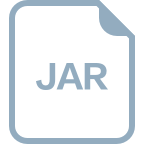
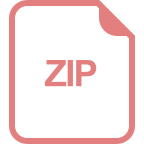






