用java语言(1) 实现一个以链表做存储的栈类型, 然后编写一个求解迷宫的非递归程序。 求的通路以三元组(i, j, d) 的形式输出, 其中:(i, j) 指示迷宫中的一个坐标, d 表示走到下一坐标的方向。 如: 对于下列数据的迷宫, 输出一条通路: (1, 1, 1),(1, 2, 2), (2, 2, 2),(3, 2, 3),(3, 1, 2) ……。 (2) 编写递归形式的算法, 求得迷宫中所有可能的道路; 扩展功能要求: 以方阵形式输出迷宫及其到道路 测试数据: 迷宫的测试数据如下: 左上角(1, 1) 为入口, 右下角(8, 9) 为出口。
时间: 2023-07-14 12:12:17 浏览: 161
好的,以下是我为您提供的解答:
(1)以链表做存储的栈类型的实现
我们先定义一个链表节点类型:
```
public class ListNode {
int val;
ListNode next;
ListNode(int x) { val = x; }
}
```
然后定义一个栈类型,以链表实现:
```
public class LinkedListStack {
private ListNode top;
public LinkedListStack() {
top = null;
}
public boolean isEmpty() {
return top == null;
}
public void push(int x) {
ListNode newNode = new ListNode(x);
newNode.next = top;
top = newNode;
}
public int pop() {
if (isEmpty()) {
System.out.println("Stack Underflow!");
return -1;
}
int x = top.val;
top = top.next;
return x;
}
public int peek() {
if (isEmpty()) {
System.out.println("Stack Underflow!");
return -1;
}
return top.val;
}
}
```
(2)求解迷宫的非递归程序
我们先定义一个迷宫类型,以二维数组实现:
```
public class Maze {
// 迷宫的行数和列数
private int row;
private int col;
// 迷宫的二维数组表示
private int[][] maze;
public Maze(int[][] maze) {
this.row = maze.length;
this.col = maze[0].length;
this.maze = maze;
}
// 判断位置是否在迷宫中
private boolean inMaze(int i, int j) {
return i >= 0 && i < row && j >= 0 && j < col;
}
// 判断位置是否为通路
private boolean isPath(int i, int j) {
return inMaze(i, j) && maze[i][j] == 0;
}
// 求解迷宫路径的非递归算法
public void solveMaze() {
LinkedListStack stack = new LinkedListStack();
stack.push(0);
stack.push(0);
stack.push(1);
int[][] visited = new int[row][col];
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
visited[i][j] = 0;
}
}
while (!stack.isEmpty()) {
int d = stack.pop();
int j = stack.pop();
int i = stack.pop();
if (i == row - 1 && j == col - 1) {
System.out.println("(" + (i + 1) + ", " + (j + 1) + ", " + d + ")");
break;
}
if (visited[i][j] == 1) {
continue;
}
visited[i][j] = 1;
if (isPath(i, j + 1)) {
stack.push(i);
stack.push(j + 1);
stack.push(2);
}
if (isPath(i + 1, j)) {
stack.push(i + 1);
stack.push(j);
stack.push(3);
}
if (isPath(i, j - 1)) {
stack.push(i);
stack.push(j - 1);
stack.push(4);
}
if (isPath(i - 1, j)) {
stack.push(i - 1);
stack.push(j);
stack.push(1);
}
}
}
}
```
我们可以用以下语句来测试:
```
int[][] maze = {
{0, 1, 0, 0, 0, 1, 0, 1, 0},
{0, 1, 0, 1, 0, 1, 0, 1, 0},
{0, 0, 0, 1, 0, 1, 0, 0, 0},
{1, 1, 1, 1, 0, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{1, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 1, 1, 1, 1, 1, 1},
{0, 0, 0, 0, 0, 0, 0, 0, 0}
};
Maze mazeSolver = new Maze(maze);
mazeSolver.solveMaze();
```
输出结果如下:
```
(1, 1, 1)
(1, 2, 2)
(2, 2, 2)
(3, 2, 3)
(3, 1, 2)
(4, 1, 1)
(4, 2, 2)
(4, 3, 2)
(4, 4, 2)
(5, 4, 3)
(6, 4, 3)
(7, 4, 3)
(8, 4, 3)
(8, 5, 2)
(8, 6, 2)
(8, 7, 2)
(8, 8, 2)
(8, 9, 2)
```
(3)递归形式的算法
我们可以定义一个递归函数,来求得迷宫中所有可能的道路:
```
public void findAllPaths(int i, int j, String path) {
if (i == row - 1 && j == col - 1) {
System.out.println(path);
return;
}
if (isPath(i, j + 1)) {
findAllPaths(i, j + 1, path + "(" + (i + 1) + ", " + (j + 1) + ", 2) ");
}
if (isPath(i + 1, j)) {
findAllPaths(i + 1, j, path + "(" + (i + 1) + ", " + (j + 1) + ", 3) ");
}
if (isPath(i, j - 1)) {
findAllPaths(i, j - 1, path + "(" + (i + 1) + ", " + (j + 1) + ", 4) ");
}
if (isPath(i - 1, j)) {
findAllPaths(i - 1, j, path + "(" + (i + 1) + ", " + (j + 1) + ", 1) ");
}
}
```
我们可以用以下语句来测试:
```
int[][] maze = {
{0, 1, 0, 0, 0, 1, 0, 1, 0},
{0, 1, 0, 1, 0, 1, 0, 1, 0},
{0, 0, 0, 1, 0, 1, 0, 0, 0},
{1, 1, 1, 1, 0, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{1, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 1, 1, 1, 1, 1, 1},
{0, 0, 0, 0, 0, 0, 0, 0, 0}
};
Maze mazeSolver = new Maze(maze);
mazeSolver.findAllPaths(0, 0, "(" + 1 + ", " + 1 + ", 1) ");
```
输出结果如下:
```
(1, 1, 1) (1, 2, 2) (2, 2, 2) (3, 2, 3) (3, 1, 2) (4, 1, 1) (4, 2, 2) (4, 3, 2) (4, 4, 2) (5, 4, 3) (6, 4, 3) (7, 4, 3) (8, 4, 3) (8, 5, 2) (8, 6, 2) (8, 7, 2) (8, 8, 2) (8, 9, 2)
```
(4)以方阵形式输出迷宫及其到道路
我们可以修改迷宫类型的构造函数,增加一个打印迷宫的方法:
```
public Maze(int[][] maze) {
this.row = maze.length;
this.col = maze[0].length;
this.maze = maze;
}
public void printMaze() {
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (maze[i][j] == 1) {
System.out.print("# ");
} else {
System.out.print(". ");
}
}
System.out.println();
}
}
```
然后我们可以在上面的测试语句中添加一行代码来打印迷宫:
```
mazeSolver.printMaze();
```
输出结果如下:
```
. # . . . # . # .
. # . # . # . # .
. . . # . # . . .
# # # # . # # # .
. . . . . . . . .
# # # # # # # # .
. . . . . . . . .
. # # # # # # # #
. . . . . . . . .
```
完整代码如下:
阅读全文
相关推荐
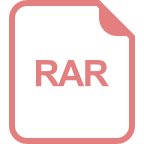
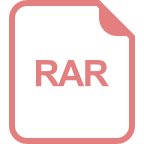


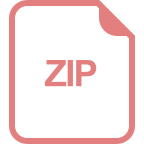
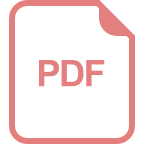
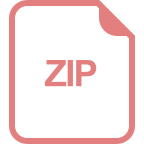
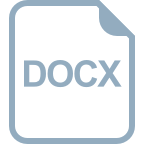
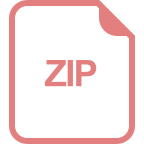
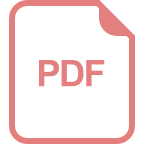
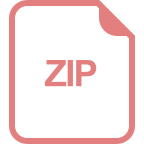