上述代码如何读取音频信号数据
时间: 2023-09-03 14:06:11 浏览: 134
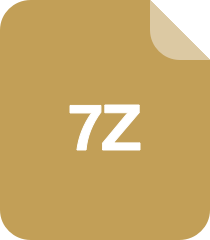
音频信号处理matlab代码

要读取音频信号数据,可以使用C语言中的文件操作函数。下面是一个简单的示例代码,演示如何从WAV文件中读取音频数据:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
char chunk_id[4];
uint32_t chunk_size;
char format[4];
char subchunk1_id[4];
uint32_t subchunk1_size;
uint16_t audio_format;
uint16_t num_channels;
uint32_t sample_rate;
uint32_t byte_rate;
uint16_t block_align;
uint16_t bits_per_sample;
char subchunk2_id[4];
uint32_t subchunk2_size;
} WavHeader;
int main()
{
FILE *fp;
WavHeader header;
short *data;
int n_samples;
// 打开WAV文件
fp = fopen("audio.wav", "rb");
if (fp == NULL) {
printf("Failed to open file.\n");
return 1;
}
// 读取WAV文件头
fread(&header, sizeof(header), 1, fp);
// 判断是否为PCM格式
if (header.audio_format != 1) {
printf("Unsupported audio format.\n");
return 1;
}
// 计算样本数
n_samples = header.subchunk2_size / header.block_align;
// 分配内存并读取音频数据
data = (short *)malloc(n_samples * sizeof(short));
fread(data, sizeof(short), n_samples, fp);
// 关闭文件
fclose(fp);
// 对音频数据进行处理,比如计算THD值
// 释放内存
free(data);
return 0;
}
```
上述代码中使用了WAV文件格式,如果你的音频数据不是WAV格式,需要根据实际情况进行修改。需要注意的是,读取音频数据时需要根据文件头中的采样率、采样位数等信息进行解析,以保证数据的正确性。
阅读全文
相关推荐
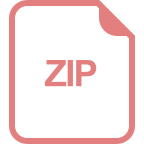
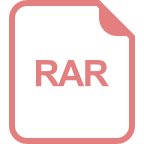















