vue3 jsx高德地图搜索功能 amap/amap-jsapi-loader 在弹窗里 ElAutocomplete
时间: 2024-02-23 13:29:56 浏览: 94
在Vue3中使用JSX和高德地图搜索功能,需要先安装amap和amap-jsapi-loader两个库,可以使用以下命令:
```
npm install amap amap-jsapi-loader
```
安装完成后,可以在Vue组件中使用ElAutocomplete组件来实现搜索功能。首先,在组件中导入所需的库:
```jsx
import { defineComponent, ref } from 'vue'
import { ElAutocomplete } from 'element-plus'
import { Loader } from '@amap/amap-jsapi-loader'
import AMap from 'amap'
```
然后,在组件的setup函数中初始化AMap和Loader,并创建一个ref来保存搜索结果:
```jsx
setup() {
const result = ref([])
const loader = new Loader({
key: 'your amap key',
version: '2.0',
plugins: []
})
const initAMap = async () => {
const AMapInstance = await loader.load()
const map = new AMapInstance.Map('map-container')
const search = new AMapInstance.PlaceSearch({
map: map,
pageSize: 10,
pageIndex: 1,
city: '全国'
})
const searchCallback = (status, result) => {
if (status === 'complete' && result.info === 'OK') {
result.value = result.poiList.pois.map((poi) => poi.name)
}
}
const handleSearch = (value) => {
search.search(value, searchCallback)
}
}
initAMap()
return {
result
}
}
```
在initAMap函数中,首先通过Loader加载AMap库和插件,然后创建一个地图和一个PlaceSearch对象,用于搜索地点。在搜索回调函数中,将搜索结果转换为一个数组,保存在result ref中。
接下来,在ElAutocomplete组件中,将result ref作为数据源,使用handleSearch函数来触发搜索:
```jsx
return {
setup() {
const result = ref([])
const loader = new Loader({
key: 'your amap key',
version: '2.0',
plugins: []
})
const initAMap = async () => {
const AMapInstance = await loader.load()
const map = new AMapInstance.Map('map-container')
const search = new AMapInstance.PlaceSearch({
map: map,
pageSize: 10,
pageIndex: 1,
city: '全国'
})
const searchCallback = (status, result) => {
if (status === 'complete' && result.info === 'OK') {
result.value = result.poiList.pois.map((poi) => poi.name)
}
}
const handleSearch = (value) => {
search.search(value, searchCallback)
}
}
initAMap()
return {
result,
handleSearch
}
},
render() {
return (
<div>
<ElAutocomplete
value={this.value}
placeholder="请输入地址"
fetchSuggestions={this.handleSearch}
popper-class="amap-autocomplete"
v-slots={{
dropdown: ({ item }) => (
<div class="amap-item">
<i class="el-icon-map-location"></i>
{item}
</div>
)
}}
onInput={(value) => (this.value = value)}
onSelect={(value) => (this.value = value)}
/>
</div>
)
}
}
```
在ElAutocomplete组件中,使用fetchSuggestions属性来绑定handleSearch函数,触发搜索操作,并将搜索结果显示在下拉列表中。同时,使用v-slots属性来自定义下拉列表中的每个选项,将搜索结果的图标和名称显示出来。在输入框的onInput和onSelect事件中,分别保存用户输入的值和选择的值。
相关推荐
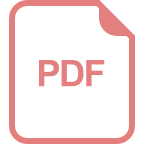
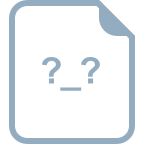
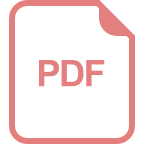














