编程题(文件操作)。定义一个学生类Student。成员有:(1)私有数据成员:ID(学号,int类型),Name(姓名,string类型)。(2)公有成员函数:GetID(),GetName()分别用于获取学生的上述信息;重载输出流运算符<<,使它能输出学生类对象的所有信息。 再编写一个主程序,主程序中定义一个对象数组,并为该对象数组赋值3个学生对象,这三个学生对象的数据可以自定。使用该输出流把这三个同学的基本信息输出到文件“sinfo1.txt”中。最后再从该文件中读取数据,把这3个学生对象信息按照学
时间: 2024-02-21 12:00:29 浏览: 23
号从小到大的顺序输出到屏幕上。
下面是代码实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
class Student {
private:
int ID;
string Name;
public:
Student(int id, string name): ID(id), Name(name) {}
int GetID() const {
return ID;
}
string GetName() const {
return Name;
}
friend ostream& operator<<(ostream& os, const Student& s) {
os << "ID: " << s.ID << ", Name: " << s.Name;
return os;
}
};
bool compare(const Student& s1, const Student& s2) {
return s1.GetID() < s2.GetID();
}
int main() {
Student students[] = {
Student(1001, "Tom"),
Student(1003, "Jerry"),
Student(1002, "Bob")
};
ofstream outfile("sinfo1.txt");
for (auto& s : students) {
outfile << s << endl;
}
outfile.close();
ifstream infile("sinfo1.txt");
string line;
Student sorted_students[3];
int i = 0;
while (getline(infile, line)) {
int id_pos = line.find("ID: ") + 4;
int name_pos = line.find(", Name: ");
int id = stoi(line.substr(id_pos, name_pos - id_pos));
string name = line.substr(name_pos + 9);
sorted_students[i++] = Student(id, name);
}
infile.close();
sort(sorted_students, sorted_students + 3, compare);
for (auto& s : sorted_students) {
cout << s << endl;
}
return 0;
}
```
程序中首先定义了一个`Student`类,其中包含一个私有的`ID`和`Name`成员变量,以及公有的`GetID()`和`GetName()`成员方法,以及一个重载输出流运算符`<<`,用于输出学生对象的信息。
然后在主程序中定义了一个学生对象数组,并通过构造函数为每个学生对象赋值。接着将这三个学生对象的信息输出到文件`sinfo1.txt`中。最后从文件中读取数据,将学生对象按照学号从小到大排序,然后输出到屏幕上。在排序时,使用了一个辅助函数`compare`,用于比较两个学生对象的学号大小。
相关推荐
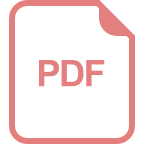
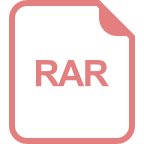
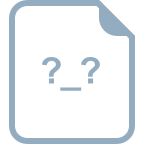














