定义一个学生类Student,含有数据成员:学号和姓名、学生人数(静态),并将数据私有化;
时间: 2024-04-29 07:24:39 浏览: 61
好的,你的要求是定义一个学生类Student,包含学号、姓名和学生人数这三个私有数据成员。我可以为你提供以下代码:
```
class Student{
private:
string id; // 学号
string name; // 姓名
static int count; // 学生人数
public:
Student(string id, string name){
this->id = id;
this->name = name;
count++;
}
string getID() const{
return id;
}
string getName() const{
return name;
}
static int getCount(){
return count;
}
};
int Student::count = 0;
```
这是一个比较简单的学生类,它包含了一个构造函数用于初始化学号和姓名,一个静态的计数器用于记录学生人数,以及三个公有的成员函数用于访问数据成员。需要注意的是,私有的数据成员不能直接从外部访问。
相关问题
定义一个学生类Student,该类有姓名、学号、年龄、专业等私有成员。除此之外,该类还有一个静态数据成员NUM。为该类定义必要的成员函数,包括构造函数和其他函数
好的,以下是一个可能的实现:
```c++
class Student {
private:
string name;
string id;
int age;
string major;
static int NUM;
public:
Student(string n, string i, int a, string m) {
name = n;
id = i;
age = a;
major = m;
NUM++;
}
string getName() const {
return name;
}
void setName(string n) {
name = n;
}
string getId() const {
return id;
}
void setId(string i) {
id = i;
}
int getAge() const {
return age;
}
void setAge(int a) {
age = a;
}
string getMajor() const {
return major;
}
void setMajor(string m) {
major = m;
}
static int getNum() {
return NUM;
}
};
```
在这个实现中,我们定义了一个名为`Student`的类,有四个私有成员,分别为`name`、`id`、`age`和`major`。其中,`NUM`是一个静态数据成员,用于记录创建的`Student`对象数量。
我们还定义了一个构造函数,可以根据传入的参数初始化这些私有成员,并且每次调用构造函数时,都会将`NUM`加1。
此外,我们还定义了一些成员函数,包括读取和设置私有成员的函数,以及一个静态函数`getNum()`,用于获取`NUM`的值。
需要注意的是,我们在类定义中只是声明了静态数据成员`NUM`,并没有为它分配内存空间。因此,我们还需要在类定义外部进行定义和初始化,如下所示:
```c++
int Student::NUM = 0;
```
这样,我们就完成了一个简单的`Student`类的定义。
c++定义并实现一个学生类(Student),类中的数据成员有:学生姓名、学生学号、人数和班级号。至少有一个公有成员函数(PrintStuInfo)。PrintStuInfo负责将相关信息输出。在类中定义静态数据成员,记录当前学生人数。在类中定义常数据成员,记录班级号,假设比较号为1001。添加一个友元函数,可对学生类中的私有数据成员进行访问。假设1001班的初始学生人数为45人。在主程序中测试新加入2个学生,并分别通过成员函数和友元函数输出输出2个学生的信息及班级总人数。
以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; // 姓名
int id; // 学号
static int num; // 学生人数
const int cls_id; // 班级号
public:
Student(string n, int i): name(n), id(i), cls_id(1001) { num++; } // 构造函数
static int getNum() { return num; } // 静态成员函数
void PrintStuInfo() // 成员函数
{
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "班级号:" << cls_id << endl;
}
friend void PrintStuInfoByFriend(Student stu); // 友元函数
};
int Student::num = 0; // 静态数据成员初始化
void PrintStuInfoByFriend(Student stu)
{
cout << "姓名:" << stu.name << endl;
cout << "学号:" << stu.id << endl;
cout << "班级号:" << stu.cls_id << endl;
}
int main()
{
Student stu1("张三", 10001);
Student stu2("李四", 10002);
cout << "学生人数:" << Student::getNum() << endl;
cout << "通过成员函数输出:" << endl;
stu1.PrintStuInfo();
stu2.PrintStuInfo();
cout << "通过友元函数输出:" << endl;
PrintStuInfoByFriend(stu1);
PrintStuInfoByFriend(stu2);
return 0;
}
```
输出结果为:
```
学生人数:2
通过成员函数输出:
姓名:张三
学号:10001
班级号:1001
姓名:李四
学号:10002
班级号:1001
通过友元函数输出:
姓名:张三
学号:10001
班级号:1001
姓名:李四
学号:10002
班级号:1001
```
相关推荐
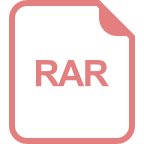
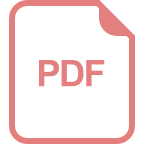
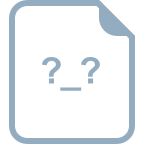












