学生参加考试,有N个学生,每个学生参加3门课程考试。要求定义学生类student,数据有学号num,姓名name,三门课程socre[3]:数学,英语,计算机;静态成员count表示学生人数。要求工程文件实现,工程名称为:自己姓名。 (1) 输入学生学号,姓名,学生成绩。(setdate 函数实现) (2) 设计构造函数和析购函数。每构造一个对象,实现个数加1,每删除一个对象,个数减1。 (3) 求每个学生的总分。(成员函数stu_sum实现) (4) 设计一个友元函数,输出学生每门课程成绩对应的等级。(友元函数kc_dj()) 90~100:优秀;80~90:良好;70~79:中等;60~69:及格;小于60:不及格。 (5) 输出学生信息,每门课程成绩,相应等级,学生总分。(print函数实现) (6) 编写主函数验证,输入学生个数,学生相应数据,输出信息。对象数组实现。用c++代码实现
时间: 2024-02-15 15:05:17 浏览: 96
以下是题目要求的代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
static int count; // 静态成员变量,记录学生人数
string num; // 学号
string name; // 姓名
int score[3]; // 三门课程成绩
public:
Student(string num, string name, int score1, int score2, int score3);
~Student();
void setdata(string num, string name, int score1, int score2, int score3);
int stu_sum();
friend void kc_dj(Student s);
void print();
};
int Student::count = 0; // 静态成员变量初始化
Student::Student(string num, string name, int score1, int score2, int score3) {
this->num = num;
this->name = name;
this->score[0] = score1;
this->score[1] = score2;
this->score[2] = score3;
count++; // 每构造一个对象,人数加1
}
Student::~Student() {
count--; // 每析构一个对象,人数减1
}
void Student::setdata(string num, string name, int score1, int score2, int score3) {
this->num = num;
this->name = name;
this->score[0] = score1;
this->score[1] = score2;
this->score[2] = score3;
}
int Student::stu_sum() {
int sum = 0;
for (int i = 0; i < 3; i++) {
sum += score[i];
}
return sum;
}
void kc_dj(Student s) {
for (int i = 0; i < 3; i++) {
int score = s.score[i];
string level;
if (score >= 90) {
level = "优秀";
} else if (score >= 80) {
level = "良好";
} else if (score >= 70) {
level = "中等";
} else if (score >= 60) {
level = "及格";
} else {
level = "不及格";
}
cout << "第" << i+1 << "门课程成绩为:" << score << ",对应等级为:" << level << endl;
}
}
void Student::print() {
cout << "学号:" << num << endl;
cout << "姓名:" << name << endl;
for (int i = 0; i < 3; i++) {
int score = this->score[i];
string level;
if (score >= 90) {
level = "优秀";
} else if (score >= 80) {
level = "良好";
} else if (score >= 70) {
level = "中等";
} else if (score >= 60) {
level = "及格";
} else {
level = "不及格";
}
cout << "第" << i+1 << "门课程成绩为:" << score << ",对应等级为:" << level << endl;
}
int sum = stu_sum();
cout << "总分为:" << sum << endl;
}
int main() {
int n;
cout << "请输入学生个数:";
cin >> n;
Student* students = new Student[n];
for (int i = 0; i < n; i++) {
string num, name;
int score1, score2, score3;
cout << "请输入第" << i+1 << "个学生的学号、姓名、数学成绩、英语成绩、计算机成绩,以空格分隔:";
cin >> num >> name >> score1 >> score2 >> score3;
students[i].setdata(num, name, score1, score2, score3);
}
for (int i = 0; i < n; i++) {
cout << "第" << i+1 << "个学生信息如下:" << endl;
students[i].print();
kc_dj(students[i]);
}
delete[] students;
return 0;
}
```
注意事项:
1. 本题要求使用对象数组来存储学生信息,需要动态申请内存,使用完毕后需要及时释放。
2. 友元函数可以访问类的私有成员,但它不是类的成员函数,所以不需要使用类名限定符。
3. 在友元函数中传入的参数为对象的副本,如果需要修改对象的成员变量,需要使用引用或指针来传参。本题中并不需要修改学生对象,所以可以直接传入副本。
阅读全文
相关推荐
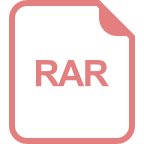
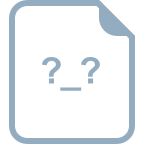















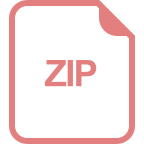
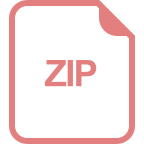