#include<iostream> #include<string> using namespace std; class TStudent { private: string id; string name; int score[3]; int totalScore; public: void Init(string id, string name, int score1,int score2, int score3) { this->id = id; this->name = name; this->score[0] = score1; this->score[1] = score2; this->score[2] = score3; this->totalScore = score1 + score2 + score3; } void show_st() { cout << "学生ID:" << id << endl; cout << "学生姓名:" << name << endl; cout << "三门学科成绩:" << score[0] << "," << score[1] << "," << score[2] << endl; cout << "总成绩:" << totalScore << endl; } int* Getscore() { return score; } }; int main() { TStudent students[5]; string id, name; int score1, score2, score3; for (int i = 0; i < 5; i++) { cout << "请输入第" << i + 1 << "个学生的信息:" << endl; cout << "学生ID:"; cin >> id; cout << "学生姓名:"; cin >> name; cout << "三门学科成绩:"; cin >> score1 >> score2 >> score3; students[i].Init(id, name, score1, score2, score3); } int totalSocre[3] = { 0 }; for (int i = 0; i < 5; i++) { int* score = students[i].Getscore(); totalScore[0] += score[0]; totalScore[1] += score[1]; totalScore[2] += score[2]; } double avgscore[3] = { 0 }; for (int i = 0; i < 3; i++) { avgscore[i] = totalScore[i] / 5.0; } for (int i = 0; i < 5; i++) { students[i].show_st(); } cout << "三门课程的平均成绩:" << avgscore[0] << " " << avgscore[1] << " " << avgscore[2] << endl; return 0; }改正
时间: 2024-01-20 11:04:46 浏览: 28
以下是修改后的代码,主要是将代码中出现的拼写错误进行了修正:
```
#include<iostream>
#include<string>
using namespace std;
class TStudent {
private:
string id;
string name;
int score[3];
int totalScore;
public:
void Init(string id, string name, int score1, int score2, int score3) {
this->id = id;
this->name = name;
this->score[0] = score1;
this->score[1] = score2;
this->score[2] = score3;
this->totalScore = score1 + score2 + score3;
}
void show_st() {
cout << "学生ID:" << id << endl;
cout << "学生姓名:" << name << endl;
cout << "三门学科成绩:" << score[0] << "," << score[1] << "," << score[2] << endl;
cout << "总成绩:" << totalScore << endl;
}
int* GetScore() {
return score;
}
};
int main() {
TStudent students[5];
string id, name;
int score1, score2, score3;
for (int i = 0; i < 5; i++) {
cout << "请输入第" << i + 1 << "个学生的信息:" << endl;
cout << "学生ID:";
cin >> id;
cout << "学生姓名:";
cin >> name;
cout << "三门学科成绩:";
cin >> score1 >> score2 >> score3;
students[i].Init(id, name, score1, score2, score3);
}
int totalScore[3] = { 0 };
for (int i = 0; i < 5; i++) {
int* score = students[i].GetScore();
totalScore[0] += score[0];
totalScore[1] += score[1];
totalScore[2] += score[2];
}
double avgScore[3] = { 0 };
for (int i = 0; i < 3; i++) {
avgScore[i] = totalScore[i] / 5.0;
}
for (int i = 0; i < 5; i++) {
students[i].show_st();
}
cout << "三门课程的平均成绩:" << avgScore[0] << " " << avgScore[1] << " " << avgScore[2] << endl;
return 0;
}
```
相关推荐
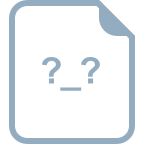
















