java宠物商店项目代码,我需要若干个宠物的类如Cat,Dog用来输入宠物的颜色,年龄,名字和出售价格。还需要一个Pet的类用来修改宠物信息,还需要用java swing来实现用户界面
时间: 2024-03-19 18:43:11 浏览: 57
好的,以下是一个简单的宠物商店项目,包括Cat、Dog和Pet类:
Cat类:
```java
public class Cat {
private String color;
private int age;
private String name;
private double price;
public Cat(String color, int age, String name, double price) {
this.color = color;
this.age = age;
this.name = name;
this.price = price;
}
public String getColor() {
return color;
}
public int getAge() {
return age;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
Dog类:
```java
public class Dog {
private String color;
private int age;
private String name;
private double price;
public Dog(String color, int age, String name, double price) {
this.color = color;
this.age = age;
this.name = name;
this.price = price;
}
public String getColor() {
return color;
}
public int getAge() {
return age;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
Pet类:
```java
public class Pet {
private String color;
private int age;
private String name;
private double price;
public Pet(String color, int age, String name, double price) {
this.color = color;
this.age = age;
this.name = name;
this.price = price;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
为了实现用户界面,我们可以使用Java Swing。以下是一个简单的GUI,允许用户添加和修改宠物的信息:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class PetShopGUI extends JFrame {
private JTextField nameField;
private JTextField ageField;
private JTextField colorField;
private JTextField priceField;
private JComboBox<String> petTypeComboBox;
private JButton addButton;
private JButton editButton;
public PetShopGUI() {
setTitle("Pet Shop");
setSize(400, 250);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints constraints = new GridBagConstraints();
constraints.fill = GridBagConstraints.HORIZONTAL;
constraints.gridx = 0;
constraints.gridy = 0;
constraints.insets = new Insets(5, 5, 5, 5);
panel.add(new JLabel("Pet Type:"), constraints);
petTypeComboBox = new JComboBox<>(new String[]{"Cat", "Dog"});
constraints.gridx = 1;
panel.add(petTypeComboBox, constraints);
constraints.gridy = 1;
panel.add(new JLabel("Name:"), constraints);
nameField = new JTextField(20);
constraints.gridx = 1;
panel.add(nameField, constraints);
constraints.gridy = 2;
panel.add(new JLabel("Age:"), constraints);
ageField = new JTextField(20);
constraints.gridx = 1;
panel.add(ageField, constraints);
constraints.gridy = 3;
panel.add(new JLabel("Color:"), constraints);
colorField = new JTextField(20);
constraints.gridx = 1;
panel.add(colorField, constraints);
constraints.gridy = 4;
panel.add(new JLabel("Price:"), constraints);
priceField = new JTextField(20);
constraints.gridx = 1;
panel.add(priceField, constraints);
addButton = new JButton("Add Pet");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String petType = (String) petTypeComboBox.getSelectedItem();
String name = nameField.getText();
int age = Integer.parseInt(ageField.getText());
String color = colorField.getText();
double price = Double.parseDouble(priceField.getText());
if (petType.equals("Cat")) {
Cat cat = new Cat(color, age, name, price);
// TODO: 添加新的猫到数据库中
} else if (petType.equals("Dog")) {
Dog dog = new Dog(color, age, name, price);
// TODO: 添加新的狗到数据库中
}
JOptionPane.showMessageDialog(null, "Pet added successfully!");
}
});
constraints.gridx = 0;
constraints.gridy = 5;
constraints.gridwidth = 2;
constraints.anchor = GridBagConstraints.CENTER;
panel.add(addButton, constraints);
editButton = new JButton("Edit Pet");
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String petType = (String) petTypeComboBox.getSelectedItem();
String name = nameField.getText();
int age = Integer.parseInt(ageField.getText());
String color = colorField.getText();
double price = Double.parseDouble(priceField.getText());
if (petType.equals("Cat")) {
Cat cat = new Cat(color, age, name, price);
// TODO: 修改数据库中的猫信息
} else if (petType.equals("Dog")) {
Dog dog = new Dog(color, age, name, price);
// TODO: 修改数据库中的狗信息
}
JOptionPane.showMessageDialog(null, "Pet updated successfully!");
}
});
constraints.gridx = 0;
constraints.gridy = 6;
constraints.gridwidth = 2;
constraints.anchor = GridBagConstraints.CENTER;
panel.add(editButton, constraints);
add(panel);
setVisible(true);
}
public static void main(String[] args) {
new PetShopGUI();
}
}
```
请注意,上述代码中的TODO注释需要根据您的具体要求进行修改,以便将新的宠物添加到数据库中并更新现有宠物的信息。
阅读全文
相关推荐
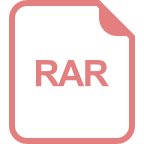

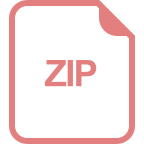

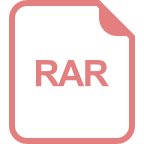
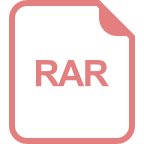
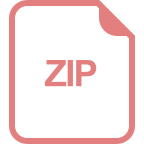
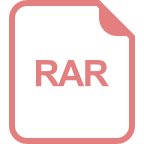
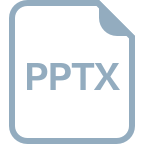







