用python计算两个经纬度之间的距离
时间: 2023-06-04 07:05:15 浏览: 759

Python库 | pygeodesic-0.1.5.tar.gz
可以使用geopy库来计算两个经纬度之间的距离。下面是一个示例代码:
```python
from geopy.distance import geodesic
coord1 = (lat1, lon1) # 第一个经纬度
coord2 = (lat2, lon2) # 第二个经纬度
distance = geodesic(coord1, coord2).km # 计算距离,单位为千米
print(f"The distance between the two coordinates is: {distance} km")
```
其中,`lat1`、`lon1`、`lat2`、`lon2`分别为两个经纬度的纬度和经度。`geodesic()`函数计算两个地点之间的大圆距离,返回结果的单位为千米。
阅读全文
相关推荐
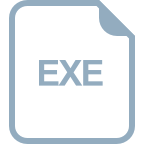
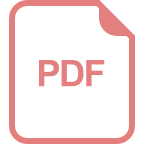





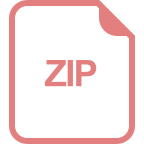
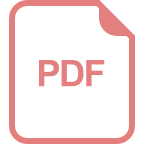
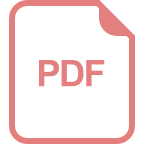





