请使用标准库函数并且使用中断处理函数实现通过USART1给stm32f407单片机发送一个数,然后能接收到10个数的程序
时间: 2023-12-03 09:45:17 浏览: 36
以下是一个示例程序,它通过USART1接收10个整数,并将它们存储在数组中:
```c
#include "stm32f4xx.h"
#define BUFFER_SIZE 10
volatile int buffer[BUFFER_SIZE];
volatile int buffer_head = 0;
volatile int buffer_tail = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) {
int data = USART_ReceiveData(USART1);
buffer[buffer_head] = data;
buffer_head = (buffer_head + 1) % BUFFER_SIZE;
}
}
int main(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// Enable clocks for USART1 and GPIOA
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// Configure USART1 TX and RX pins
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Connect USART1 TX and RX pins to AF7 (USART1)
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_USART1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_USART1);
// Configure USART1
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1 RX interrupt
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// Configure NVIC for USART1
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
// Wait for 10 numbers to be received
while (buffer_tail != BUFFER_SIZE) {}
// Print the received numbers
for (int i = 0; i < BUFFER_SIZE; i++) {
printf("%d\n", buffer[i]);
}
while (1) {}
return 0;
}
```
此程序使用了中断处理函数来处理USART1接收到的数据。当有一个字符接收到USART1时,中断处理函数将该字符存储在数组中,并将`buffer_head`指针向前移动。在主循环中,我们等待`buffer_tail`指针移动到`BUFFER_SIZE`。一旦所有数字都被接收并存储在数组中,我们打印它们并进入无限循环。
相关推荐
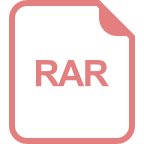
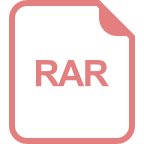














