在VS中用C语言中创建一个结点数为i(用户输入)的链表,要求利用循环结构,创建链表单独定义一个函数
时间: 2023-08-04 15:35:00 浏览: 82
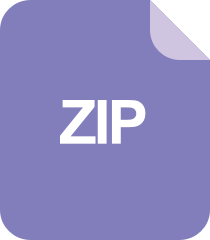
通过C语言实现数据结构的循环链表
好的,下面是用 C 语言创建一个结点数为 i 的链表的代码,需要先定义链表节点的结构体:
```c
struct ListNode {
int val;
struct ListNode* next;
};
```
然后,编写一个函数来创建一个指定长度的链表:
```c
struct ListNode* createLinkedList(int n) {
struct ListNode* head = NULL;
struct ListNode* cur = NULL;
for (int i = 1; i <= n; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = i;
node->next = NULL;
if (head == NULL) {
head = node;
cur = node;
} else {
cur->next = node;
cur = node;
}
}
return head;
}
```
在这个函数中,我们首先定义了一个链表头节点的指针 `head` 和一个当前节点的指针 `cur`,然后使用循环结构来创建链表节点,并将它们连接起来。
最后,我们返回链表头节点的指针,即可得到一个长度为 `n` 的链表。
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode* next;
};
struct ListNode* createLinkedList(int n) {
struct ListNode* head = NULL;
struct ListNode* cur = NULL;
for (int i = 1; i <= n; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = i;
node->next = NULL;
if (head == NULL) {
head = node;
cur = node;
} else {
cur->next = node;
cur = node;
}
}
return head;
}
int main() {
int n;
printf("请输入链表的长度:");
scanf("%d", &n);
struct ListNode* head = createLinkedList(n);
printf("链表的值为:");
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
return 0;
}
```
希望能帮到你!
阅读全文
相关推荐
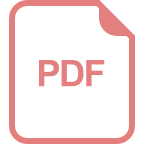
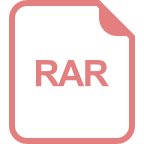
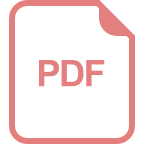
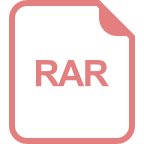
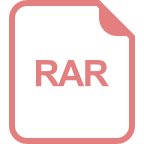
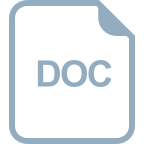
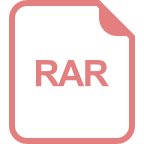
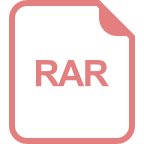
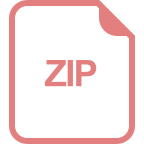
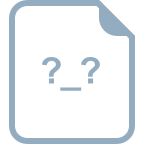
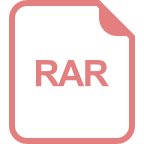
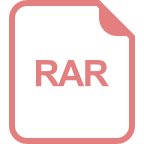
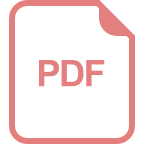
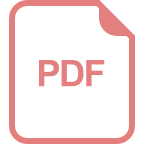
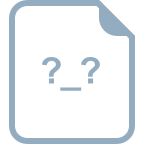
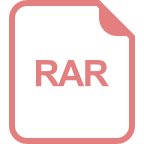