用C++代码:使用模板类对给定的查找集合顺序查找与给定值k相等的元素,对给定的查找集合折半查找与给定值k相等的元素
时间: 2023-11-27 14:49:16 浏览: 68
顺序查找:
```c++
#include <iostream>
using namespace std;
template <class T>
int sequentialSearch(T arr[], int n, T key) {
for (int i = 0; i < n; i++) {
if (arr[i] == key) {
return i;
}
}
return -1;
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
int key = 3;
int index = sequentialSearch(arr, n, key);
if (index != -1) {
cout << "Element found at index " << index << endl;
} else {
cout << "Element not found" << endl;
}
return 0;
}
```
折半查找:
```c++
#include <iostream>
using namespace std;
template <class T>
int binarySearch(T arr[], int n, T key) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == key) {
return mid;
} else if (arr[mid] < key) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
int key = 3;
int index = binarySearch(arr, n, key);
if (index != -1) {
cout << "Element found at index " << index << endl;
} else {
cout << "Element not found" << endl;
}
return 0;
}
```
阅读全文
相关推荐
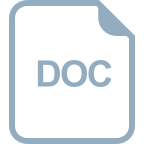
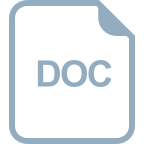
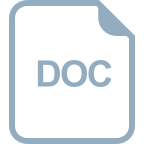






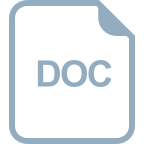
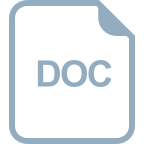
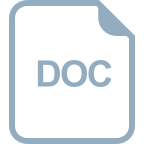
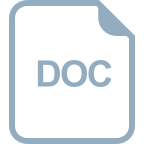
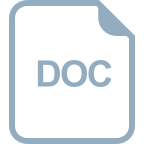



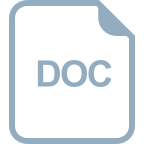
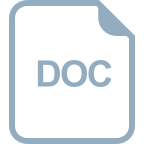
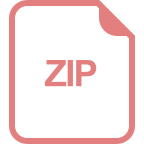