写一个C++词法分析器,从源程序文件中读入字符,统计行数和列数用于错误单词的定位,删除空格类字符,包括回车、制表符空格,按拼写单词,并用(内码,属性)二元式表示。(属性值——token 的机内表示)
时间: 2024-05-06 18:18:39 浏览: 17
以下是一个简单的C++词法分析器示例代码,可以读取文件中的字符并进行基本的词法分析,输出对应的(内码,属性)二元组:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
#include <cctype>
using namespace std;
// 定义Token结构体
struct Token {
int code; // 内码
int attribute; // 属性值
int line; // 行号
int column; // 列号
};
// 判断字符是否为字母或数字
bool isAlnum(char c) {
return isalpha(c) || isdigit(c);
}
// 词法分析函数
vector<Token> lex(string filename) {
vector<Token> tokens; // 存储二元组的向量
map<string, int> keywords = {{"if", 1}, {"else", 2}, {"while", 3}, {"do", 4}, {"break", 5}}; // 关键词表
ifstream file(filename); // 打开文件
if (!file.is_open()) {
cout << "Error opening file!" << endl;
return tokens;
}
char c; // 当前字符
int line = 1, column = 0; // 行号和列号
while (file.get(c)) {
column++;
if (c == '\n') {
line++;
column = 0;
} else if (isspace(c)) {
continue; // 忽略空格类字符
} else if (isAlnum(c)) {
string word;
word += c;
while (file.get(c) && isAlnum(c)) {
word += c;
column++;
}
file.unget(); // 将多读的一个字符放回流中
// 判断是否为关键词
if (keywords.count(word)) {
tokens.push_back(Token{keywords[word], 0, line, column - word.length() + 1});
} else {
tokens.push_back(Token{0, 0, line, column - word.length() + 1});
}
} else {
tokens.push_back(Token{c, 0, line, column}); // 其他符号直接作为内码
}
}
file.close();
return tokens;
}
int main() {
string filename = "test.txt";
vector<Token> tokens = lex(filename);
for (auto token : tokens) {
cout << "(" << token.code << ", " << token.attribute << ") at (" << token.line << ", " << token.column << ")" << endl;
}
return 0;
}
```
需要注意的是,该代码仅实现了基本的词法分析功能,并未考虑更复杂的情况(如注释、字符串字面量等),在实际应用中还需根据需要进行修改和完善。
相关推荐
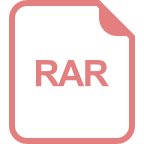
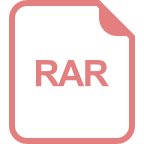
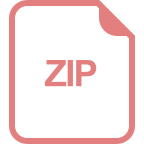














