#include <stdio.h> #include<string.h> #include<stdlib.h> void copy_int(int val, void* buf, int maxbytes) { if (maxbytes - (int)sizeof(val) >= 0) memcpy(buf, (void*)&val, sizeof(val)); } int main() { int max = 1114; void* buff = malloc(max); copy_int(514, &buff, 114); int a = *(int*)buff; printf("%d", a); }这段代码有没有错误
时间: 2023-11-20 13:04:44 浏览: 46
这段代码有一个错误,就是在调用 `copy_int` 函数时,传递的第二个参数应该是 `buff` 而不是 `&buff`。因为 `buff` 已经是一个指向 void 类型的指针,不需要再取其地址。修改后的代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void copy_int(int val, void* buf, int maxbytes) {
if (maxbytes - (int)sizeof(val) >= 0)
memcpy(buf, (void*)&val, sizeof(val));
}
int main() {
int max = 1114;
void* buff = malloc(max);
copy_int(514, buff, 114);
int a = *(int*)buff;
printf("%d", a);
free(buff); // 释放内存
return 0;
}
```
注意,还需要在程序结束时释放 `buff` 所分配的内存,避免内存泄漏。
相关问题
#include<stdio.h> #include<stdlib.h>
#include<stdio.h>和#include<stdlib.h>是C语言中的预处理指令,用于引入标准输入输出函数和动态内存分配函数。它们通常一起使用,但有时候会出现报错的情况。
报错的原因可能是由于编译器或IDE的设置问题,或者是代码中的其他错误导致的。在某些情况下,删除#include<stdlib.h>可能会解决报错问题,但这并不是一个通用的解决方案。
为了解决这个问题,你可以尝试以下几个步骤:
1. 确保你的编译器或IDE已正确配置,并且能够找到正确的头文件路径。
2. 检查你的代码中是否存在其他错误,例如语法错误、函数声明错误等。
3. 尝试使用不同的编译器或IDE来编译你的代码,看是否能够解决问题。
4. 如果以上步骤都没有解决问题,你可以尝试在代码中手动声明所需的函数,而不使用#include<stdlib.h>。例如,你可以在代码中添加以下声明:
```c
void *malloc(size_t size);
void free(void *ptr);
```
请注意,这只是一种临时解决方案,如果你的代码依赖于stdlib.h中的其他函数或定义,可能会导致其他问题。
#include <stdio.h> #include <stdlib.h>
#include <stdio.h>和#include <stdlib.h>是C语言中的两个头文件。
1. #include <stdio.h>是用来包含标准输入输出函数的头文件。它提供了一些用于输入和输出的函数,比如printf和scanf等。通过包含这个头文件,我们可以在程序中使用这些函数来进行输入和输出操作。
2. #include <stdlib.h>是用来包含标准库函数的头文件。它提供了一些常用的函数,比如内存分配函数malloc和free,字符串转换函数atoi和atof等。通过包含这个头文件,我们可以在程序中使用这些函数来进行各种操作。
相关推荐
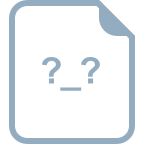
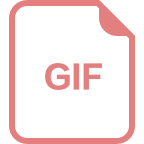
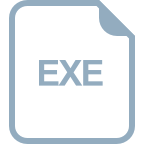
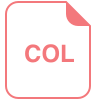
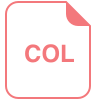











