Vue创建一个子组件,子组件内容为 一个input标签和一个按钮,当单 击按钮时会去检查input标签中内容是否为空,如果为空,则弹窗提示内容为空
时间: 2023-08-31 07:25:48 浏览: 86
### 回答1:
这是一个技术问题,我可以回答。您需要在子组件的模板中加入一个input标签和一个按钮,给按钮添加一个click事件。
在点击事件中,先通过$refs访问input标签,获取它的值。然后判断是否为空,如果为空,可以使用element-ui等UI库弹出提示框,提醒用户输入内容。
具体实现可以参照以下代码:
<template>
<div>
<input ref="inputContent" />
<button @click="checkInput">检查内容</button>
</div>
</template>
<script>
export default {
methods: {
checkInput() {
const content = this.$refs.inputContent.value
if (!content || content.trim() === '') {
this.$message.error('内容不能为空')
return
}
// do something
}
}
}
</script>
### 回答2:
在Vue中创建一个子组件,可以通过以下步骤实现:
1. 在父组件中使用子组件:
```html
<template>
<div>
<!-- 其他内容 -->
<child-component></child-component>
</div>
</template>
```
2. 在子组件中定义input标签和按钮:
```html
<template>
<div>
<input type="text" v-model="inputValue" />
<button @click="checkInput">检查内容</button>
</div>
</template>
```
3. 在子组件的data选项中定义inputValue变量,并在方法中定义checkInput方法:
```javascript
<script>
export default {
data() {
return {
inputValue: ''
}
},
methods: {
checkInput() {
if (this.inputValue.trim() === '') {
alert('内容不能为空');
}
}
}
}
</script>
```
这样,当点击按钮时,子组件会检查input标签中的内容是否为空,如果为空,则弹窗提示内容为空。
### 回答3:
可以通过Vue的组件和事件绑定来实现创建一个子组件,该子组件包含一个input标签和一个按钮,并且在按钮点击时检查input标签中的内容是否为空,如果为空则弹窗提示内容为空。
首先,在父组件中引入子组件,并通过v-model来实现与子组件input标签的双向绑定。代码如下:
```vue
// 父组件
<template>
<div>
<child-component v-model="inputValue"></child-component>
<button @click="checkInput">检查</button>
</div>
</template>
<script>
import childComponent from './childComponent.vue'
export default {
components: {
childComponent
},
data() {
return {
inputValue: ''
}
},
methods: {
checkInput() {
if (this.inputValue === '') {
alert('内容为空!')
}
}
}
}
</script>
```
然后,在子组件中使用props接收父组件的value,并通过v-model将input标签与父组件的value进行绑定。代码如下:
```vue
// 子组件 childComponent.vue
<template>
<div>
<input type="text" v-model="inputValue" />
<button>按钮</button>
</div>
</template>
<script>
export default {
props: ['value'],
data() {
return {
inputValue: this.value
}
},
watch: {
inputValue() {
this.$emit('input', this.inputValue)
}
}
}
</script>
```
在父组件中点击按钮时,会调用checkInput方法进行输入内容的检查,如果内容为空,则弹窗提示内容为空。
注意:以上代码仅为示例,具体的实现方式可能因项目框架和需求而有所不同。
阅读全文
相关推荐
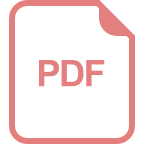

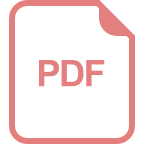
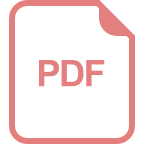
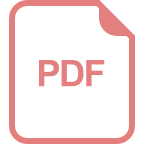
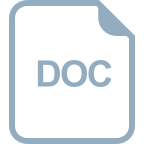
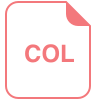
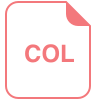
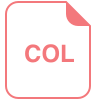
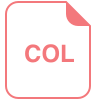
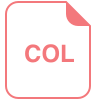
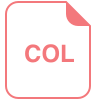
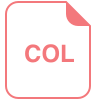
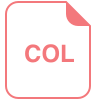
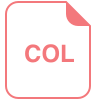
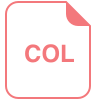