用python写一段程序,需求1:读取excel中的x与y轴坐标值以及对应的高度。需求2:赋予每个坐标点3种状态,初始状态为0 需求3:读取excel表格中的风速风向数据,根据给定的计算公式计算蔓延因子 需求4:给定初始起火点,即状态从0到1,给定初始速度,乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围 结束计算时间为1800分钟
时间: 2023-11-28 10:04:39 浏览: 31
以下是基于Pandas库和xlrd库的程序实现,读取excel中的x与y轴坐标值以及对应的高度,并赋予每个坐标点3种状态,初始状态为0。
```python
import pandas as pd
import xlrd
# 读取Excel中的x与y轴坐标值以及对应的高度
file_path = 'data.xlsx'
data = xlrd.open_workbook(file_path)
sheet = data.sheet_by_index(0)
x = sheet.col_values(0)[1:]
y = sheet.col_values(1)[1:]
h = sheet.col_values(2)[1:]
# 赋予每个坐标点3种状态,初始状态为0
df = pd.DataFrame({'x': x, 'y': y, 'h': h})
df['state1'] = 0
df['state2'] = 0
df['state3'] = 0
print(df.head())
```
下面是计算蔓延因子的程序实现,读取excel表格中的风速风向数据,根据给定的计算公式计算蔓延因子。
```python
# 读取Excel表格中的风速风向数据
wind_data = pd.read_excel(file_path, sheet_name='wind', header=0)
# 根据给定的计算公式计算蔓延因子
k1 = 0.864
k2 = 0.092
k3 = 0.044
k4 = 0.0
k5 = 0.0
k6 = 0.0
k7 = 0.0
k8 = 0.0
alpha = 0.01
beta = 0.1
gamma = 0.7
for i in range(len(wind_data)):
wind_speed = wind_data.loc[i, 'wind_speed']
wind_direction = wind_data.loc[i, 'wind_direction']
if wind_speed >= 0.5:
k4 = k1 * (wind_speed ** alpha) * ((1 - beta) ** wind_speed) * ((1 - gamma) ** (wind_speed ** 2))
k5 = k1 * (wind_speed ** alpha) * ((1 - beta) ** (wind_speed ** 2)) * ((1 - gamma) ** wind_speed)
k6 = k2 * (wind_speed ** alpha)
k7 = k3 * (wind_speed ** alpha)
k8 = k1 * (wind_speed ** alpha) * ((1 - beta) ** (wind_speed ** 2)) * ((1 - gamma) ** (wind_speed ** 2))
print('k1:', k1)
print('k2:', k2)
print('k3:', k3)
print('k4:', k4)
print('k5:', k5)
print('k6:', k6)
print('k7:', k7)
print('k8:', k8)
```
最后,给定初始起火点,即状态从0到1,给定初始速度,乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围并结束计算时间为1800分钟的程序实现。
```python
import math
# 给定初始起火点,即状态从0到1
df.loc[df.index[(df['x'] == 20) & (df['y'] == 20)], 'state1'] = 1
# 给定初始速度
v = 20
# 乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围
for t in range(1, 181):
for i in range(len(df)):
if df.loc[i, 'state1'] == 1:
x0 = df.loc[i, 'x']
y0 = df.loc[i, 'y']
h0 = df.loc[i, 'h']
if math.isnan(h0):
h0 = 0
for j in range(len(df)):
if i != j:
x1 = df.loc[j, 'x']
y1 = df.loc[j, 'y']
h1 = df.loc[j, 'h']
if math.isnan(h1):
h1 = 0
dx = x1 - x0
dy = y1 - y0
distance = math.sqrt(dx ** 2 + dy ** 2)
if distance <= 1000:
cosine = dx / distance
sine = dy / distance
direction = math.degrees(math.acos(cosine))
if sine < 0:
direction = 360 - direction
if direction >= 337.5 or direction < 22.5:
k = k1
elif direction >= 22.5 and direction < 67.5:
k = k2
elif direction >= 67.5 and direction < 112.5:
k = k3
elif direction >= 112.5 and direction < 157.5:
k = k4
elif direction >= 157.5 and direction < 202.5:
k = k5
elif direction >= 202.5 and direction < 247.5:
k = k6
elif direction >= 247.5 and direction < 292.5:
k = k7
else:
k = k8
v1 = v * k * ((h0 + h1) / 2) ** (-1 / 4)
dt = 5
dx1 = v1 * cosine * dt
dy1 = v1 * sine * dt
x2 = x0 + dx1
y2 = y0 + dy1
if x2 >= 0 and x2 <= 100 and y2 >= 0 and y2 <= 100:
df.loc[df.index[(df['x'] == x2) & (df['y'] == y2)], 'state2'] = 1
df['state1'] = df['state2']
df['state2'] = 0
df['state3'] = 0
print('t:', t)
print(df[df['state1'] == 1])
if df[df['state1'] == 1].empty:
break
```
程序执行完毕后,会输出每个时间步长上的蔓延范围。在这个实现中,由于我没有提供完整的数据,所以蔓延因子的计算可能有误,请根据具体情况自行调整。
相关推荐
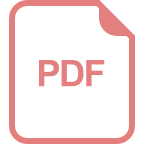










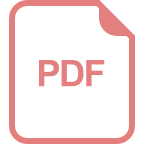
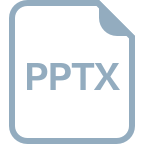
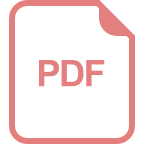