从键盘输入两个点的坐标x1.y1.x2.y2,然后输出他们之间的距离
时间: 2024-10-09 21:00:49 浏览: 29
两点之间距离的计算通常使用毕达哥拉斯定理,即直角三角形斜边长度等于两直角边平方和的平方根。假设第一个点的坐标为(x1, y1),第二个点的坐标为(x2, y2),那么它们之间的距离d可以使用下面的公式计算:
```python
import math
def calculate_distance(x1, y1, x2, y2):
distance = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
return distance
# 用户输入两个点的坐标
x1, y1 = map(int, input("请输入第一个点的坐标(x1,y1),例如:4,5:").split(','))
x2, y2 = map(int, input("请输入第二个点的坐标(x2,y2),例如:6,8:").split(','))
# 计算并打印两点之间的距离
distance = calculate_distance(x1, y1, x2, y2)
print(f"两点 ({x1}, {y1}) 和 ({x2}, {y2}) 之间的距离是 {distance}")
相关问题
要求从键盘输入两个点的坐标 x1、y1、x2、y2,然后输出它们之间的距离的C语言
以下是计算两个点之间距离的C语言程序:
```c
#include <stdio.h>
#include <math.h>
int main() {
double x1, y1, x2, y2, distance;
printf("Enter the coordinates of the first point (x1, y1): ");
scanf("%lf %lf", &x1, &y1);
printf("Enter the coordinates of the second point (x2, y2): ");
scanf("%lf %lf", &x2, &y2);
distance = sqrt(pow((x2-x1), 2) + pow((y2-y1), 2));
printf("The distance between the two points is: %lf\n", distance);
return 0;
}
```
程序要求用户输入两个点的坐标,然后使用数学库函数`sqrt()`和`pow()`计算两个点之间的距离,并输出结果。
编写程序,从键盘输入两点的坐标(x1,y1)和(x2,y2),计算并输出两点之间的距离。 要求: 输入格式:依次输入 4 个数字作为 x1、y1、x2、y2 的值。 输出格式:如样例输出计算结果,结果保留 2 位小数。
下面是一个 Python 的实现:
```python
import math
x1, y1, x2, y2 = map(float, input().split())
distance = math.sqrt((x1-x2)**2 + (y1-y2)**2)
print('%.2f' % distance)
```
首先从输入中读取四个浮点数,分别赋值给 x1、y1、x2、y2。然后使用数学库中的 sqrt 函数计算两点之间的距离,并输出结果。由于要求结果保留两位小数,因此使用格式化输出控制输出的小数位数。
阅读全文
相关推荐
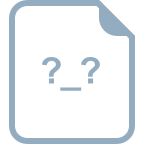
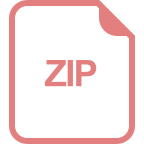
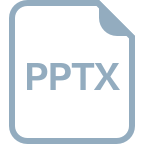







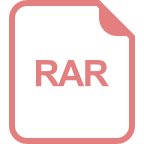
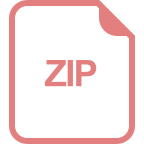
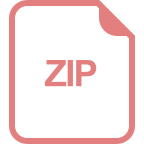
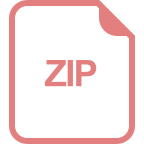
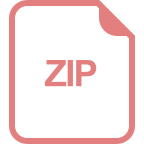
