如果要比较我新写入的结构体字符串与文件中已有结构体字符串是否重复该怎么办,举例给出代码
时间: 2024-05-11 08:16:26 浏览: 7
您好,根据您的要求,以下是一段 C++ 代码,用于比较已有结构体字符串和新写入结构体字符串是否重复:
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义结构体
struct Student {
string name;
int age;
string gender;
};
// 读取文件中已有的结构体字符串
bool readFile(Student s) {
ifstream file("students.txt");
string line;
while (getline(file, line)) {
// 按照格式解析字符串
size_t found = line.find("|");
string name = line.substr(0, found);
line = line.substr(found + 1);
found = line.find("|");
int age = stoi(line.substr(0, found));
line = line.substr(found + 1);
string gender = line;
// 比较结构体对象的属性是否相等
if (name == s.name && age == s.age && gender == s.gender) {
file.close();
return true;
}
}
file.close();
return false;
}
// 将新写入的结构体字符串写入文件
void writeFile(Student s) {
ofstream file("students.txt", ios::app);
file << s.name << "|" << s.age << "|" << s.gender << endl;
file.close();
}
int main() {
// 新写入的结构体对象
Student newS = {"John", 20, "Male"};
// 检查是否重复
if (readFile(newS)) {
cout << "Error: Duplicate record found!" << endl;
} else {
// 写入文件
writeFile(newS);
cout << "Record added successfully!" << endl;
}
return 0;
}
```
在此示例代码中,我们首先定义了一个`Student`结构体,包含`name`、`age`和`gender`三个属性。然后,`readFile()`函数用于读取文件`students.txt`中的已有结构体字符串,并逐一比较每个结构体对象的属性是否相等,如果有重复则返回`true`,否则返回`false`。`writeFile()`函数用于将新写入的结构体字符串写入文件。在`main()`函数中,我们定义了一个新的`Student`结构体对象`newS`,然后调用`readFile()`函数进行检查,如果有重复则输出错误信息,否则调用`writeFile()`函数将新结构体字符串写入文件。
希望这段代码能够帮助您解决问题。如果您有任何疑问,请随时与我联系。
相关推荐
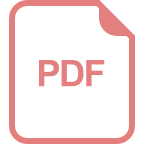
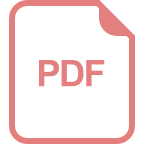
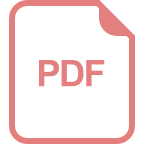
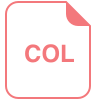
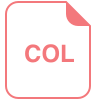
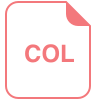
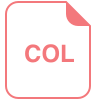
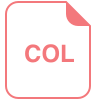









