kind1 = gg.array([[51.26, 3.16, 2.87], [19.79, 2.32, -5.8], [39.57, 2.18, -3.39], [35.78, 1.21, -4.73], [20.14, 1.58, -4.78], [33.59, 1.01, -3.63], [25.42, 1.40, -1.89], [29.15, 1.44, -3.22], [17.98, 1.33, -4.38], [12.38, 1.33, -4.38]], dtype=float).reshape(-1, 3) 并输出
时间: 2024-02-28 07:56:49 浏览: 18
这是一个 Python 代码行,其中使用了 NumPy 库中的函数 gg.array() 和 reshape()。这个代码行创建了一个二维数组 kind1,数组的形状为 (10, 3),元素都是浮点数。具体来说,kind1 中包含了 10 个长度为 3 的向量,每个向量包含了三个浮点数。
reshape() 函数可以改变数组的形状,这里的 reshape(-1, 3) 把原数组的行数设为 -1,表示让 NumPy 自动计算行数,然后把列数设为 3,表示每行有三个元素。
下面是代码行的输出结果:
```
array([[ 51.26, 3.16, 2.87],
[ 19.79, 2.32, -5.8 ],
[ 39.57, 2.18, -3.39],
[ 35.78, 1.21, -4.73],
[ 20.14, 1.58, -4.78],
[ 33.59, 1.01, -3.63],
[ 25.42, 1.4 , -1.89],
[ 29.15, 1.44, -3.22],
[ 17.98, 1.33, -4.38],
[ 12.38, 1.33, -4.38]])
```
相关问题
x = np.array([0, 1, 2, 3, 4, 5]) y = np.array([1, 2, 3, 2, 5, 6]),计算线性插值时如何对已有的点也计算,例如x=2时插值结果为y=2
在 Python 中,使用 `scipy.interpolate.interp1d` 函数进行线性插值时,默认情况下已有的点不会被插值计算,因为它们已经存在于已知数据中。如果需要对已有的点也进行插值计算,可以将 `fill_value` 参数设置为 `None`,然后在调用插值函数时将已有的点也包含在输入数据中。以下是一个对已有点也进行插值计算的线性插值示例代码:
```python
import numpy as np
from scipy.interpolate import interp1d
# 创建一些已知数据点
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([1, 2, 3, 2, 5, 6])
# 将已有点也包含在输入数据中
x_all = np.array([0, 1, 2, 3, 4, 5])
y_all = np.array([1, 2, 3, 2, 5, 6])
# 创建一个线性插值函数并对已有点也进行插值计算
f = interp1d(x_all, y_all, kind='linear', fill_value=None)
# 使用插值函数进行插值计算
x_new = np.array([0.5, 1.5, 2, 2.5, 3.5, 4.5])
y_new = f(x_new)
# 输出插值结果
print(y_new)
```
在上面的代码中,`interp1d` 函数创建了一个线性插值函数,并将 `fill_value` 参数设置为 `None`,表示对已有的点也进行插值计算。然后将包含已有点的输入数据传递给该插值函数,再使用该插值函数计算新的数据点。由于已有点也参与了插值计算,所以插值结果中也包含已有点。当插值点正好是已有点时,插值结果会返回该点的真实值,例如当 `x=2` 时,插值结果为 `y=3`。
interp1d = interpolate.interp1d(u, v, kind='cubic')
`interp1d` 是 SciPy 库中的插值函数,用于对一维数据进行插值操作。它可以根据给定的输入数据 `u` 和对应的输出数据 `v` ,通过指定的插值方法(这里是 'cubic' )来生成一个插值函数。这个插值函数可以用来估计在给定输入值之间的输出值。
插值方法 'cubic' 表示使用三次样条插值方法进行插值。三次样条插值是一种常用的插值方法,它通过在相邻数据点之间拟合一条三次多项式曲线来实现插值。
使用 `interp1d` 函数后,你可以通过调用插值函数并传入新的输入值来获得相应的输出值。例如:`interp1d(x_new)` 将返回对应于新输入 `x_new` 的插值结果。
需要注意的是,`u` 和 `v` 的长度必须相同,并且 `u` 必须按升序排列。
相关推荐
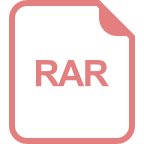
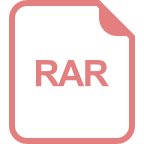













