用C语言程序做一个飞机大战游戏
时间: 2024-01-13 15:04:45 浏览: 77
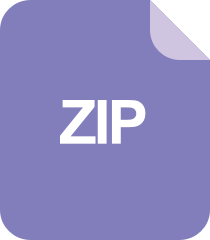
游戏开发 c语言 程序设计 飞机大战
好的,下面是一个简单的飞机大战游戏的C语言程序,你可以根据自己的需求进行修改和优化。
```c
#include <stdio.h>
#include <conio.h>
#include <windows.h>
#include <time.h>
#define WIDTH 40
#define HEIGHT 20
int score = 0;
void setCursorPosition(int x, int y) {
COORD position = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), position);
}
void hideCursor() {
CONSOLE_CURSOR_INFO cursor_info = {1, 0};
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
}
void drawBorder() {
for (int i = 0; i < WIDTH + 2; ++i) {
setCursorPosition(i, 0);
printf("#");
setCursorPosition(i, HEIGHT + 1);
printf("#");
}
for (int i = 0; i < HEIGHT + 2; ++i) {
setCursorPosition(0, i);
printf("#");
setCursorPosition(WIDTH + 1, i);
printf("#");
}
}
void drawPlane(int x, int y) {
setCursorPosition(x, y);
printf(" /\\ ");
setCursorPosition(x, y + 1);
printf(" / \\ ");
setCursorPosition(x, y + 2);
printf("/____\\");
}
void drawBullet(int x, int y) {
setCursorPosition(x, y);
printf("*");
}
void drawEnemy(int x, int y) {
setCursorPosition(x, y);
printf(" | ");
setCursorPosition(x, y + 1);
printf("\\_o_/");
setCursorPosition(x, y + 2);
printf(" / \\ ");
}
void updateScore() {
setCursorPosition(WIDTH + 4, 3);
printf("Score: %d", score);
}
int main() {
hideCursor();
drawBorder();
drawPlane(WIDTH / 2 - 3, HEIGHT - 3);
int planeX = WIDTH / 2 - 3;
int bulletX = -1;
int bulletY = -1;
int enemyX = -1;
int enemyY = 1;
srand((unsigned) time(NULL));
while (1) {
Sleep(50);
// Move plane
if (kbhit()) {
char c = getch();
if (c == 'a' && planeX > 1) {
drawPlane(planeX, HEIGHT - 3);
planeX--;
}
if (c == 'd' && planeX < WIDTH - 7) {
drawPlane(planeX, HEIGHT - 3);
planeX++;
}
if (c == ' ') {
if (bulletY == -1) {
bulletX = planeX + 3;
bulletY = HEIGHT - 4;
drawBullet(bulletX, bulletY);
}
}
}
// Move bullet
if (bulletY > 0) {
drawBullet(bulletX, bulletY);
bulletY--;
if (bulletY == 0) {
drawBullet(bulletX, bulletY);
bulletY = -1;
}
}
// Move enemy
if (enemyX == -1 && enemyY == -1) {
enemyX = rand() % (WIDTH - 4) + 2;
enemyY = 1;
drawEnemy(enemyX, enemyY);
} else if (enemyY < HEIGHT) {
drawEnemy(enemyX, enemyY);
enemyY++;
if (bulletX != -1 && bulletY == enemyY && bulletX >= enemyX && bulletX <= enemyX + 4) {
drawBullet(bulletX, bulletY);
bulletY = -1;
enemyX = -1;
enemyY = -1;
score++;
updateScore();
}
if (enemyY == HEIGHT) {
drawEnemy(enemyX, enemyY);
enemyX = -1;
enemyY = -1;
}
}
}
return 0;
}
```
这个程序使用了Windows控制台API来实现游戏界面和控制游戏元素的移动。通过键盘输入来控制飞机的移动和发射子弹,敌机会自动向下移动,当子弹击中敌机时,得分加一。
阅读全文
相关推荐
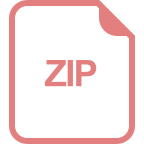
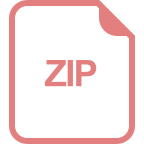













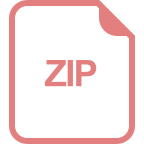
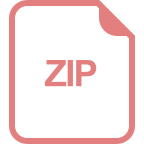
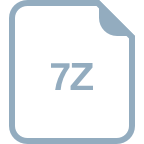