如何在QRect上面显示鼠标轨迹
时间: 2023-08-31 18:20:36 浏览: 47
### 回答1:
要在QRect上显示鼠标轨迹,您需要执行以下步骤:
1. 创建一个继承自QWidget的新类,这个新类将承载您的绘图代码。
2. 在这个新类中,重写mouseMoveEvent函数,以便跟踪鼠标的位置,并在QRect上显示轨迹。例如,您可以将鼠标位置存储在一个列表中,并在绘图函数中使用这个列表绘制轨迹。
以下是一个简单的示例,演示如何实现这个功能:
```python
from PyQt5.QtWidgets import QWidget, QApplication
from PyQt5.QtGui import QPainter, QPen, QColor, QBrush, QMouseEvent
from PyQt5.QtCore import QPoint, QRect
class MouseTrackWidget(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.mouse_positions = []
def mouseMoveEvent(self, event: QMouseEvent):
pos = event.pos()
self.mouse_positions.append(pos)
self.update()
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
# 绘制矩形
rect = QRect(50, 50, 200, 200)
pen = QPen(QColor("#000000"))
brush = QBrush(QColor("#ffffff"))
painter.setPen(pen)
painter.setBrush(brush)
painter.drawRect(rect)
# 绘制鼠标轨迹
pen = QPen(QColor("#ff0000"))
pen.setWidth(3)
painter.setPen(pen)
for pos in self.mouse_positions:
painter.drawPoint(pos)
if __name__ == "__main__":
app = QApplication([])
widget = MouseTrackWidget()
widget.show()
app.exec_()
```
在这个示例中,我们创建了一个MouseTrackWidget类,继承自QWidget。我们在这个类中定义了mouse_positions列表,它用于存储鼠标位置。我们重写了mouseMoveEvent函数,以便将每个鼠标位置添加到mouse_positions列表中。我们还重写了paintEvent函数,以便绘制矩形和鼠标轨迹。在paintEvent函数中,我们使用QPainter来绘制矩形和轨迹。我们使用drawRect函数绘制矩形,并使用drawPoint函数绘制鼠标轨迹。
在主程序中,我们创建了一个QApplication实例,并实例化了MouseTrackWidget类。最后,我们显示了窗口,并启动了应用程序的事件循环。
### 回答2:
要在QRect上显示鼠标轨迹,可以按照以下步骤进行操作:
1. 创建一个QRect实例,用于表示鼠标轨迹区域。
2. 在QRect上重新实现鼠标事件的处理函数,包括鼠标移动、按下和释放等事件。
3. 在鼠标移动事件中,获取到当前鼠标位置,并将其添加到一个用于存储鼠标轨迹点的数据结构中,比如QVector或std::vector。
4. 在绘制事件中,使用QPainter等工具,根据存储的鼠标轨迹点,绘制轨迹线或其他想要显示的效果。
5. 在鼠标释放事件中,清空存储鼠标轨迹点的数据结构,以准备接收下一次的鼠标轨迹。
下面是一个简单的示例代码,演示如何通过QRect显示鼠标轨迹:
```cpp
#include <QApplication>
#include <QRect>
#include <QKeyEvent>
#include <QMouseEvent>
#include <QPainter>
class CustomRect : public QRect
{
public:
CustomRect() : QRect() {}
protected:
QVector<QPoint> mouseTrail;
void mouseMoveEvent(QMouseEvent* event) override
{
mouseTrail.append(event->pos());
update();
}
void paintEvent(QPaintEvent *event) override
{
QRect::paintEvent(event);
QPainter painter(this);
painter.setPen(Qt::red);
for (const auto& point : mouseTrail)
{
painter.drawPoint(point);
}
}
void mouseReleaseEvent(QMouseEvent* event) override
{
mouseTrail.clear();
update();
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
CustomRect rect;
rect.setGeometry(100, 100, 300, 200);
rect.show();
return app.exec();
}
```
以上代码定义了一个自定义的QRect类CustomRect,我们可以通过继承QRect类,重新实现鼠标事件的处理函数,从而实现在QRect上显示鼠标轨迹的效果。在这个例子中,鼠标移动时会将鼠标位置添加到mouseTrail向量中,在绘制事件中,使用红色画笔逐个绘制鼠标轨迹点,而在鼠标释放事件中,清空mouseTrail向量。
### 回答3:
要在QRect上显示鼠标轨迹,首先需要通过监控鼠标事件来获取鼠标的位置信息。可以使用Qt提供的鼠标事件过滤器来实现这一功能。
具体步骤如下:
1. 定义一个QWidget或者QMainWindow的子类,作为应用程序的主窗口。
2. 重写主窗口类中的eventFilter函数,该函数用于监控鼠标事件。
3. 在eventFilter函数中,通过QEvent参数判断当前事件的类型,如果是鼠标移动事件(QEvent::MouseMove),则获取鼠标的位置信息,并在QRect上绘制轨迹。
4. 在主窗口的构造函数中调用installEventFilter函数,并将自身作为参数传入,以安装鼠标事件过滤器。
下面是一个简单的示例代码:
```cpp
#include <QtWidgets>
class MainWindow : public QMainWindow
{
public:
MainWindow(QWidget *parent = nullptr) : QMainWindow(parent),
{
installEventFilter(this);
}
protected:
bool eventFilter(QObject *watched, QEvent *event) override
{
if (event->type() == QEvent::MouseMove) {
QMouseEvent *mouseEvent = static_cast<QMouseEvent *>(event);
QPoint mousePos = mouseEvent->pos();
// 在QRect上绘制鼠标轨迹
QPainter painter(this);
painter.drawRect(QRect(mousePos.x(), mousePos.y(), 1, 1)); // 绘制1x1大小的点作为轨迹
return true;
}
return QMainWindow::eventFilter(watched, event);
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
```
通过以上步骤,我们可以在QRect上显示鼠标轨迹。每次鼠标移动时,都会在QRect上绘制一个小点,从而形成鼠标轨迹的效果。
相关推荐
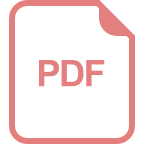
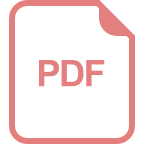
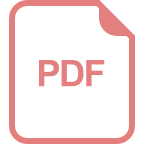














