C语言递归,计算游戏人员年龄
时间: 2024-10-27 17:08:26 浏览: 40
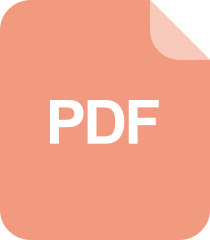
C语言中递归调用的教学设计.pdf
C语言中的递归是指函数在其定义中直接或间接地调用自己的过程。对于计算游戏人员年龄的递归场景,假设我们有一个简单的规则:如果今年是闰年,那么2月出生的人就比实际年龄小一岁;如果不是闰年,则按照常规计算。我们可以编写一个递归函数来解决这个问题:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 判断是否为闰年的条件:能被4整除且不是100的倍数,或者是400的倍数
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0))
return 1; // 闰年
else
return 0; // 非闰年
}
int calculate_age(int current_year, int birth_month, int birth_day) {
if (current_year - birth_year <= 0) { // 如果当前年份小于或等于出生年份
return 0; // 返回0,表示年龄还没到
} else if (birth_month == 2 && is_leap_year(current_year)) {
// 如果出生月份为2月且是闰年,减去1
return current_year - birth_year - 1;
} else {
// 其他情况,直接减去出生年份
return current_year - birth_year;
}
}
int main() {
int birth_year, birth_month, birth_day;
printf("请输入您的出生年、月、日:");
scanf("%d %d %d", &birth_year, &birth_month, &birth_day);
int current_year = 2023; // 假设当前是2023年
int age = calculate_age(current_year, birth_month, birth_day);
printf("您的理论年龄(考虑闰年规则)是:%d岁\n", age);
return 0;
}
```
阅读全文
相关推荐
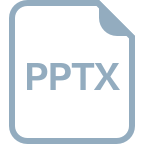
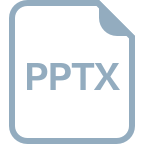
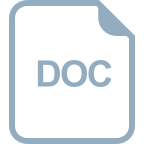
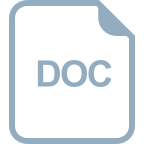
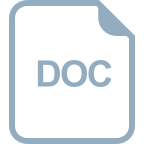
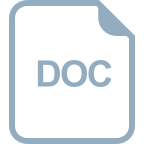
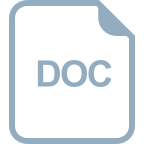
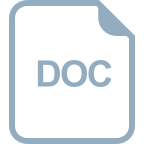
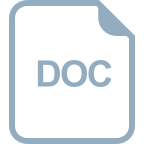
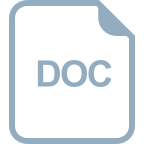
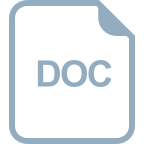
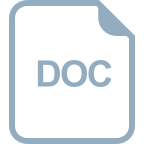
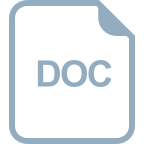
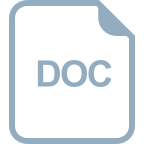
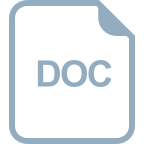
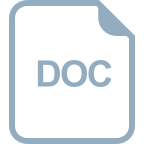
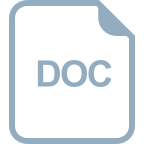
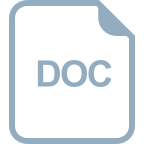
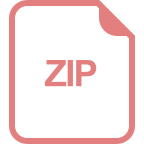