四连通种子填充算法代码c
时间: 2024-04-14 07:24:14 浏览: 160
四连通种子填充算法是一种图像处理算法,用于将指定的种子点周围的相邻像素填充为指定的颜色。以下是一个简单的C语言实现示例:
```c
#include <stdio.h>
#define WIDTH 10
#define HEIGHT 10
// 定义图像数据
int image[WIDTH][HEIGHT] = {
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 0},
{0, 0, 1, 0, 0, 0, 1, 1, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
};
// 四连通种子填充算法
void seedFill(int x, int y, int targetColor, int replacementColor) {
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) {
return;
}
if (image[x][y] != targetColor) {
return;
}
image[x][y] = replacementColor;
seedFill(x + 1, y, targetColor, replacementColor);
seedFill(x - 1, y, targetColor, replacementColor);
seedFill(x, y + 1, targetColor, replacementColor);
seedFill(x, y - 1, targetColor, replacementColor);
}
int main() {
int seedX = 5;
int seedY = 5;
int targetColor = 1;
int replacementColor = 2;
printf("原始图像:\n");
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
printf("%d ", image[i][j]);
}
printf("\n");
}
seedFill(seedX, seedY, targetColor, replacementColor);
printf("填充后的图像:\n");
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
printf("%d ", image[i][j]);
}
printf("\n");
}
return 0;
}
```
这段代码中,我们定义了一个10x10的图像数据,其中1表示目标颜色,0表示其他颜色。`seedFill`函数用于进行四连通种子填充,通过递归调用自身来填充相邻的像素。`main`函数中指定了种子点的坐标、目标颜色和替换颜色,并打印出填充前后的图像数据。
阅读全文
相关推荐
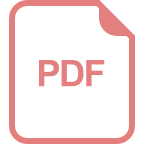
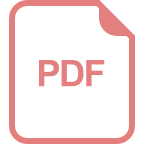
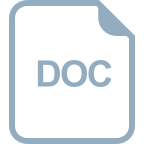
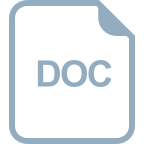
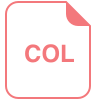

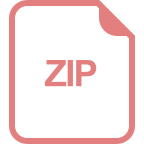
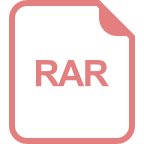
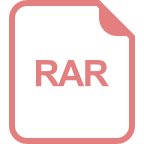
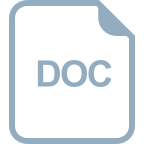
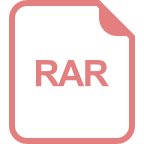
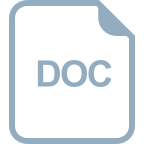
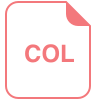
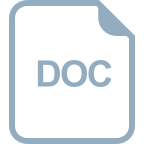
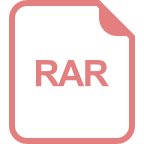
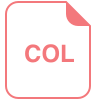