图算法:掌握基础,探索图论世界(附算法实战应用)
发布时间: 2024-07-20 00:14:12 阅读量: 27 订阅数: 27 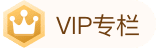
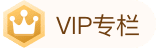
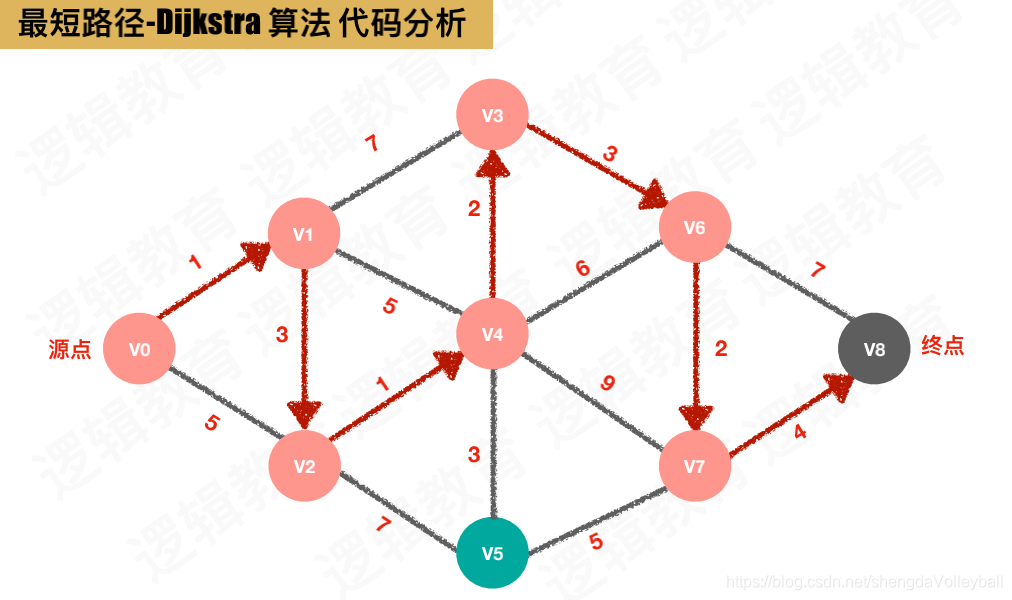
# 1. 图论基础
图论是研究图结构及其性质的数学分支。图是一个由顶点和边组成的结构,其中顶点表示实体,边表示实体之间的关系。图论在计算机科学、运筹学和社会科学等领域有着广泛的应用。
图论的基本概念包括:
- **顶点:**图中的基本元素,表示实体。
- **边:**连接两个顶点的线段,表示实体之间的关系。
- **度:**一个顶点连接的边的数量。
- **权重:**边上附加的一个值,表示边的强度或重要性。
- **路径:**连接两个顶点的顶点序列。
- **环:**一条从一个顶点开始和结束的路径。
# 2. 图论算法基础
图论算法是用于处理图结构数据的一种算法。图是一种数据结构,它由一组节点和连接这些节点的边组成。图论算法可以用于解决各种问题,例如查找最短路径、最小生成树和最大匹配。
### 2.1 图论算法的分类
图论算法可以分为两大类:
- **深度优先搜索(DFS)**:DFS 从一个节点开始,并沿着该节点的边递归地遍历图。DFS 用于查找图中的环和连通分量。
- **广度优先搜索(BFS)**:BFS 从一个节点开始,并按层级遍历图。BFS 用于查找图中的最短路径和最小生成树。
### 2.1.1 深度优先搜索(DFS)
DFS 的算法流程如下:
1. 从一个节点开始,并将其标记为已访问。
2. 遍历该节点的所有未访问的邻接节点。
3. 对每个未访问的邻接节点,重复步骤 1 和 2。
4. 当所有节点都被访问后,算法结束。
DFS 的时间复杂度为 O(V + E),其中 V 是图中的节点数,E 是图中的边数。
### 2.1.2 广度优先搜索(BFS)
BFS 的算法流程如下:
1. 从一个节点开始,并将其添加到队列中。
2. 从队列中取出一个节点,并将其标记为已访问。
3. 遍历该节点的所有未访问的邻接节点,并将它们添加到队列中。
4. 重复步骤 2 和 3,直到队列为空。
BFS 的时间复杂度也为 O(V + E)。
### 2.2 图论算法的复杂度分析
图论算法的复杂度主要由图的大小和算法的类型决定。
### 2.2.1 时间复杂度
图论算法的时间复杂度通常与图的大小成正比。例如,DFS 和 BFS 的时间复杂度都为 O(V + E)。
### 2.2.2 空间复杂度
图论算法的空间复杂度通常与图的大小成正比。例如,DFS 和 BFS 的空间复杂度都为 O(V)。
### 代码示例
以下代码示例演示了 DFS 和 BFS 算法:
```python
# DFS 算法
def dfs(graph, start):
visited = set()
stack = [start]
while stack:
node = stack.pop()
if node not in visited:
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
stack.append(neighbor)
# BFS 算法
def bfs(graph, start):
visited = set()
queue = [start]
while queue:
node = queue.pop(0)
if node not in visited:
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
queue.append(neighbor)
```
# 3. 图论算法实战
### 3.1 最小生成树算法
最小生成树(MST)算法旨在找到图中连接所有顶点的最小权重子图,该子图是一棵树。MST 在网络设计、聚类和优化等领域有着广泛的应用。
#### 3.1.1 普里姆算法
普里姆算法是一种贪心算法,从一个顶点开始,逐步添加权重最小的边,直到所有顶点都被连接。算法步骤如下:
```python
def prim(graph):
# 初始化最小生成树
mst = set()
# 选择一个顶点作为起点
start_vertex = next(iter(graph.vertices))
mst.add(start_vertex)
# 循环添加权重最小的边
while len(mst) < len(graph.vertices):
# 找到连接 MST 和非 MST 顶点的权重最小的边
min_edge = None
for vertex in mst:
for edge in graph.edges[vertex]:
if edge.to not in mst and (min_edge is None or edge.weight < min_edge.weight):
min_edge = edge
# 将权重最小的边添加到 MST 中
mst.add(min_edge.to)
```
**逻辑分析:**
* 算法从一个顶点开始,逐步添加权重最小的边。
* 每一步,算法都会找到连接 MST 和非 MST 顶点的权重最小的边。
* 算法重复此过程,直到所有顶点都被连接。
**参数说明:**
* `graph`:要查找 MST 的图。
#### 3.1.2 克鲁斯卡尔算法
克鲁斯卡尔算法也是一种贪心算法,但它使用不同的策略。算法步骤如下:
```python
def kruskal(graph):
# 初始化并查集
disjoint_set = DisjointSet()
for vertex in graph.vertices:
disjoint_set.make_set(vertex)
# 按权重从小到大对边排序
edges = sorted(graph.edges, key=lambda edge: edge.weight)
# 循环添加边
for edge in edges:
# 如果边的两个顶点不在同一个集合中,则添加边
if disjoint_set.find_set(edge.from) != disjoint_set.find_set(edge.to):
disjoint_set.union(edge.from, edge.to)
yield edge
```
**逻辑分析:**
* 算法首先对边按权重从小到大排序。
* 然后,算法循环遍历边,并检查边的两个顶点是否不在同一个集合中。
* 如果不在,则添加边并更新并查集。
**参数说明:**
* `graph`:要查找 MST 的图。
### 3.2 最短路径算法
最短路径算法旨在找到图中两个顶点之间的最短路径。最短路径算法在导航、物流和网络优化等领域有着广泛的应用。
#### 3.2.1 迪杰斯特拉算法
迪杰斯特拉算法是一种贪心算法,从一个顶点开始,逐步扩展最短路径,直到找到目标顶点。算法步骤如下:
```python
def dijkstra(graph, start_vertex, target_vertex):
# 初始化距离和父节点
distances = {vertex: float('inf') for vertex in graph.vertices}
distances[start_vertex] = 0
parents = {vertex: None for vertex in graph.vertices}
# 初始化优先队列
pq = PriorityQueue()
pq.put(start_vertex, 0)
# 循环遍历优先队列
while not pq.empty():
# 获取距离最小的顶点
current_vertex = pq.get()
# 如果当前顶点是目标顶点,则停止
if current_vertex == target_vertex:
break
# 遍历当前顶点的相邻顶点
for edge in graph.edges[current_vertex]:
# 计算到相邻顶点的距离
distance = distances[current_vertex] + edge.weight
# 如果到相邻顶点的距离更短,则更新距离和父节点
if distance < distances[edge.to]:
distances[edge.to] = distance
parents[edge.to] = current_vertex
pq.put(edge.to, distance)
# 返回最短路径
path = []
current_vertex = target_ver
```
0
0
相关推荐
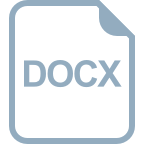
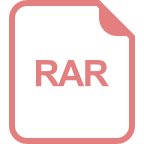
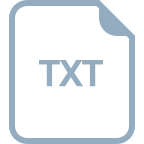





