请写出八皇后问题的java代码
时间: 2024-04-29 22:26:38 浏览: 96
以下是八皇后问题的Java代码:
```java
public class EightQueensProblem {
static int N = 8;
static int[] board = new int[N];
public static void main(String[] args) {
solve(0);
}
public static boolean canPlace(int rowIndex, int colIndex) {
// check if there is already a queen in the same column
for (int i = 0; i < rowIndex; i++) {
if (board[i] == colIndex)
return false;
}
// check if there is already a queen in the diagonal
for (int i = 0; i < rowIndex; i++) {
if (rowIndex - i == Math.abs(colIndex - board[i]))
return false;
}
return true;
}
public static boolean solve(int row) {
if (row == N) {
// print the solution
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (board[i] == j)
System.out.print("Q ");
else
System.out.print(". ");
}
System.out.println("");
}
return true;
}
for (int i = 0; i < N; i++) {
if (canPlace(row, i)) {
board[row] = i;
if (solve(row + 1))
return true;
}
}
return false;
}
}
```
该代码使用回溯算法解决了八皇后问题,使用 board 数组来存储每行放置的皇后所在的列号。canPlace 函数用于检测在 (rowIndex, colIndex) 位置放置皇后是否合法。solve 函数使用递归来依次从第 0 行到第 7 行放置皇后,如果成功找到了一组解,则输出并返回 true,否则返回 false。
阅读全文
相关推荐
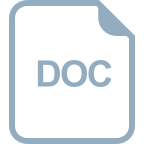
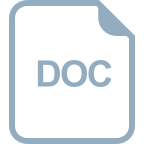
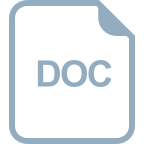



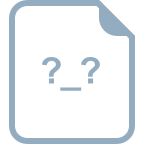
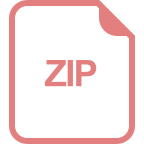
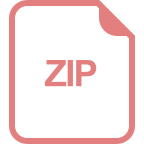
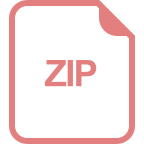
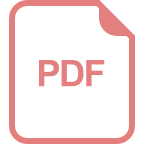
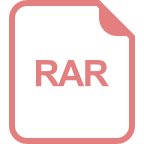
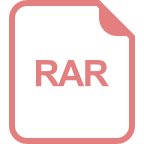
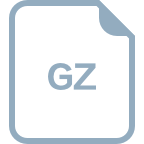
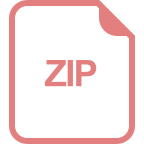
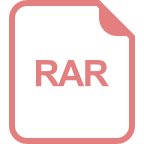
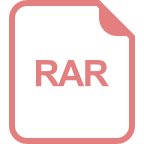
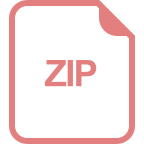