用Java语言实现Trie树的算法设计
时间: 2023-02-25 19:56:06 浏览: 94
Trie树是一种树形结构,通常用于存储字符串,并快速查找字符串是否存在。
在Java语言中,可以使用一个类来实现Trie树,该类包含两个主要部分:节点类和Trie树类。
节点类包含:
1. 字母
2. 标志,用于指示该节点是否为字符串的末尾
3. 孩子数组,用于存储下一个字母的位置
Trie树类包含:
1. 根节点
2. 插入方法,用于向Trie树中插入字符串
3. 查询方法,用于查询字符串是否存在
4. 删除方法,用于删除字符串
下面是一个简单的Java实现:
```
class TrieNode {
char c;
boolean isLeaf;
TrieNode[] children = new TrieNode[26];
public TrieNode() {}
public TrieNode(char c){
this.c = c;
}
}
class Trie {
private TrieNode root;
public Trie() {
root = new TrieNode();
}
public void insert(String word) {
TrieNode p = root;
for (int i = 0; i < word.length(); i++) {
char c = word.charAt(i);
int index = c - 'a';
if (p.children[index] == null) {
TrieNode temp = new TrieNode(c);
p.children[index] = temp;
p = temp;
} else {
p = p.children[index];
}
}
p.isLeaf = true;
}
public boolean search(String word) {
TrieNode p = searchNode(word);
if (p == null) {
return false;
} else {
if (p.isLeaf)
return true;
}
return false;
}
public boolean startsWith(String prefix) {
TrieNode p = searchNode(prefix);
if (p == null) {
阅读全文
相关推荐
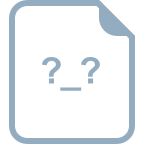
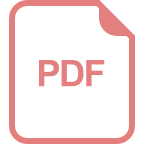


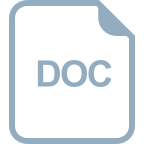
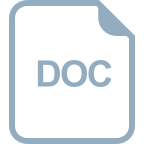
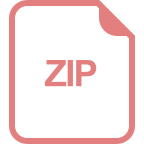
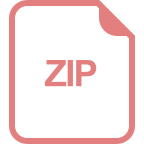
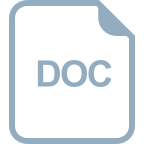
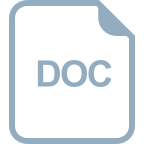
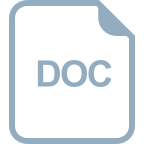
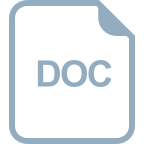
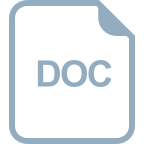
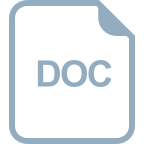
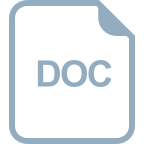
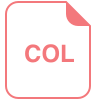
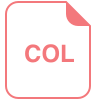