scrapy pipeline用法
时间: 2024-01-21 07:03:12 浏览: 128
Scrapy Pipeline是Scrapy框架中的一个组件,它用于在爬虫数据爬取完毕后对数据进行处理,例如数据清洗、去重、存储等操作。Pipeline是Scrapy的一个重要部分,它可以帮助我们对爬虫获取的数据进行预处理,从而提高数据质量和数据处理效率。
下面是Scrapy Pipeline的使用步骤:
1. 创建一个Pipeline类,继承自scrapy的Pipeline类。该类负责对爬虫获取到的数据进行处理。
```python
import scrapy
from scrapy.exceptions import DropItem
class MyPipeline(object):
def process_item(self, item, spider):
# 数据处理代码
return item
```
2. 在settings.py文件中设置Pipeline。可以设置多个Pipeline,它们会按照顺序依次处理数据。在这里,我们只设置一个Pipeline:
```python
ITEM_PIPELINES = {
'myproject.pipelines.MyPipeline': 300,
}
```
这里的`300`是Pipeline的优先级,数字越小,优先级越高。
3. 在Pipeline中实现数据处理逻辑。在上面的示例代码中,我们实现了一个简单的数据清洗逻辑。
4. 在Spider中生成Item并交给Pipeline处理。在Spider中,我们可以通过`yield`关键字将获取到的数据生成`Item`对象,并交给Pipeline处理:
```python
import scrapy
class MySpider(scrapy.Spider):
name = 'example.com'
allowed_domains = ['example.com']
start_urls = ['http://www.example.com']
def parse(self, response):
for sel in response.xpath('//ul/li'):
item = MyItem()
item['title'] = sel.xpath('a/text()').extract_first()
item['link'] = sel.xpath('a/@href').extract_first()
item['desc'] = sel.xpath('text()').extract_first()
yield item
```
这里的`MyItem`是我们在Spider中定义的数据结构,它包含了我们需要获取的数据。
5. Pipeline处理完成后的数据存储。在Pipeline中,我们可以将处理完成的数据存储到数据库、文件或者其他存储介质中。下面是一个将数据存储到MongoDB数据库中的示例:
```python
import pymongo
class MyPipeline(object):
def __init__(self, mongo_uri, mongo_db):
self.mongo_uri = mongo_uri
self.mongo_db = mongo_db
@classmethod
def from_crawler(cls, crawler):
return cls(
mongo_uri=crawler.settings.get('MONGO_URI'),
mongo_db=crawler.settings.get('MONGO_DATABASE', 'items')
)
def open_spider(self, spider):
self.client = pymongo.MongoClient(self.mongo_uri)
self.db = self.client[self.mongo_db]
def close_spider(self, spider):
self.client.close()
def process_item(self, item, spider):
collection = self.db[spider.name]
collection.insert_one(dict(item))
return item
```
在上面的代码中,我们使用了MongoDB来存储处理完成后的数据。需要注意的是,在Pipeline中,我们需要定义`open_spider`和`close_spider`方法,在Spider开始和结束时分别调用它们,从而保证数据处理的完整性。
以上就是Scrapy Pipeline的基本用法,它可以帮助我们对爬虫获取到的数据进行预处理,从而提高数据处理效率。
阅读全文
相关推荐
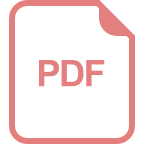
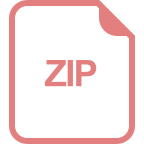


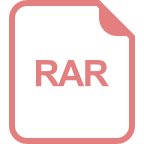
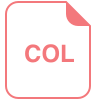
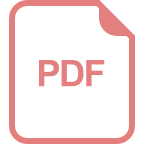
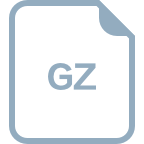
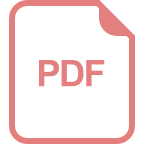
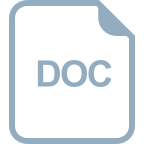
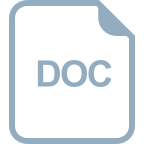
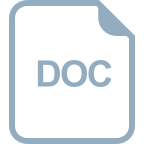
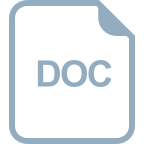
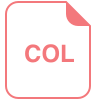
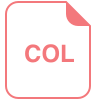

