java创建银行卡类,并且设计银行卡的两个子类储蓄卡和信用卡
时间: 2024-11-03 12:16:16 浏览: 45
在Java中,我们可以首先创建一个名为`BankAccount`的基类,它代表所有类型的银行卡。然后,可以创建两个子类`SavingsCard`和`CreditCard`来体现具体的储蓄卡和信用卡特性。这里是一个简单的例子:
```java
// 银行卡基类
public abstract class BankAccount {
private String accountNumber;
private String holderName;
private double balance;
// 构造函数
public BankAccount(String accountNumber, String holderName) {
this.accountNumber = accountNumber;
this.holderName = holderName;
this.balance = 0.0;
}
// 抽象方法,用于存款和取款操作
public abstract void deposit(double amount);
public abstract void withdraw(double amount);
// 公共方法获取信息
public String getAccountNumber() { return accountNumber; }
public String getHolderName() { return holderName; }
public double getBalance() { return balance; }
}
// 储蓄卡子类
public class SavingsCard extends BankAccount {
// 存储额外的信息,如利息率
private double interestRate;
public SavingsCard(String accountNumber, String holderName, double initialBalance) {
super(accountNumber, holderName, initialBalance);
this.interestRate = 0.05; // 例如年利率为5%
}
@Override
public void deposit(double amount) {
super.deposit(amount);
balance += amount * interestRate; // 计算并更新余额
}
@Override
public void withdraw(double amount) {
if (amount <= balance) {
super.withdraw(amount);
balance -= amount;
} else {
System.out.println("Insufficient funds.");
}
}
}
// 信用卡子类
public class CreditCard extends BankAccount {
private int creditLimit;
private boolean isOverLimit;
public CreditCard(String accountNumber, String holderName, double initialBalance, int limit) {
super(accountNumber, holderName, initialBalance);
creditLimit = limit;
isOverLimit = false;
}
@Override
public void deposit(double amount) {
if (amount > 0) {
super.deposit(amount);
if (getBalance() + amount > creditLimit) {
isOverLimit = true;
}
}
}
@Override
public void withdraw(double amount) {
if (!isOverLimit && amount <= getBalance()) {
super.withdraw(amount);
} else {
System.out.println("Withdrawal denied due to credit limit or insufficient funds.");
}
}
}
// 示例用法
public static void main(String[] args) {
SavingsCard savings = new SavingsCard("123456", "Alice", 1000.0);
savings.deposit(500.0);
System.out.println(savings.getBalance());
CreditCard credit = new CreditCard("789012", "Bob", 5000.0, 6000.0);
credit.withdraw(2000.0);
System.out.println(credit.isOverLimit());
}
```
阅读全文
相关推荐
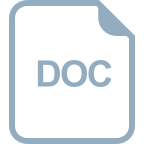
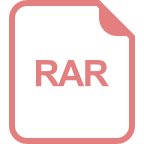
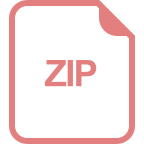

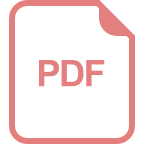
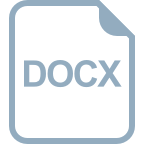
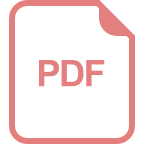
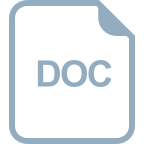
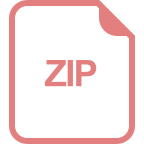
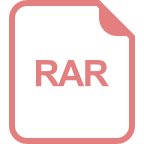
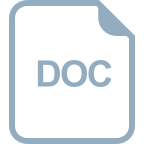
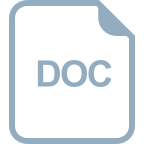
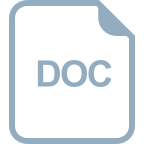


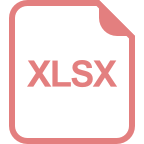
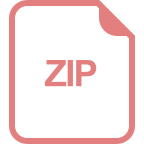
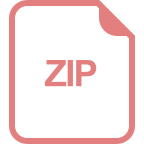
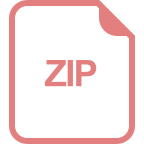
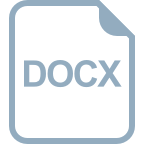