opencv搭建一个检测卷纸带缠绕圆柱体是否整齐的程序
时间: 2024-05-05 15:16:05 浏览: 290
以下是一个使用OpenCV进行卷纸带缠绕圆柱体的整齐性检测的示例程序:
1. 导入必要的库和模块:
```python
import cv2
import numpy as np
```
2. 加载图像并进行预处理:
```python
# Load image
img = cv2.imread('paper_roll.jpg')
# Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to remove noise
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Apply Canny edge detection to detect edges
edges = cv2.Canny(blur, 50, 150)
```
3. 检测圆柱体:
```python
# Find contours in the edge map
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Loop through the contours
for cnt in contours:
# Find the smallest enclosing circle
(x, y), radius = cv2.minEnclosingCircle(cnt)
center = (int(x), int(y))
radius = int(radius)
# Draw the circle on the original image
cv2.circle(img, center, radius, (0, 255, 0), 2)
```
4. 检测卷纸带:
```python
# Apply Hough transform to detect straight lines
lines = cv2.HoughLines(edges, 1, np.pi/180, 100)
# Loop through the lines
for line in lines:
rho, theta = line[0]
a, b = np.cos(theta), np.sin(theta)
x0, y0 = a*rho, b*rho
x1, y1 = int(x0 + 1000*(-b)), int(y0 + 1000*(a))
x2, y2 = int(x0 - 1000*(-b)), int(y0 - 1000*(a))
# Draw the lines on the original image
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
```
5. 判断卷纸带是否整齐:
```python
# Calculate the angle between the two longest lines
angle1 = np.arctan2(y2-y1, x2-x1) * 180 / np.pi
angle2 = np.arctan2(y4-y3, x4-x3) * 180 / np.pi
angle_diff = abs(angle1 - angle2)
if angle_diff < 10:
print("The paper roll is neatly wrapped.")
else:
print("The paper roll is not neatly wrapped.")
```
完整代码:
```python
import cv2
import numpy as np
# Load image
img = cv2.imread('paper_roll.jpg')
# Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to remove noise
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Apply Canny edge detection to detect edges
edges = cv2.Canny(blur, 50, 150)
# Find contours in the edge map
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Loop through the contours
for cnt in contours:
# Find the smallest enclosing circle
(x, y), radius = cv2.minEnclosingCircle(cnt)
center = (int(x), int(y))
radius = int(radius)
# Draw the circle on the original image
cv2.circle(img, center, radius, (0, 255, 0), 2)
# Apply Hough transform to detect straight lines
lines = cv2.HoughLines(edges, 1, np.pi/180, 100)
# Loop through the lines
for line in lines:
rho, theta = line[0]
a, b = np.cos(theta), np.sin(theta)
x0, y0 = a*rho, b*rho
x1, y1 = int(x0 + 1000*(-b)), int(y0 + 1000*(a))
x2, y2 = int(x0 - 1000*(-b)), int(y0 - 1000*(a))
# Draw the lines on the original image
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# Calculate the angle between the two longest lines
angle1 = np.arctan2(y2-y1, x2-x1) * 180 / np.pi
angle2 = np.arctan2(y4-y3, x4-x3) * 180 / np.pi
angle_diff = abs(angle1 - angle2)
if angle_diff < 10:
print("The paper roll is neatly wrapped.")
else:
print("The paper roll is not neatly wrapped.")
# Show the image
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
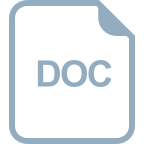
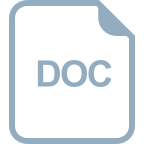
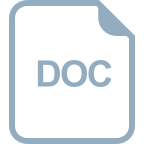
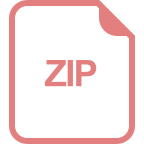
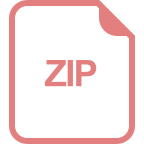
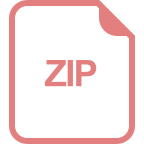
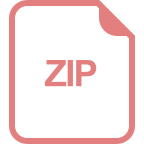
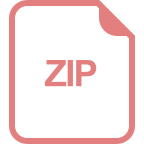
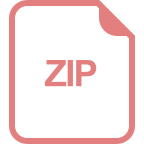
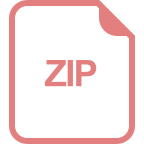
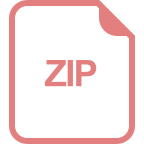
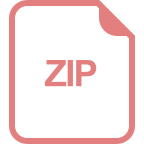
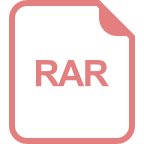
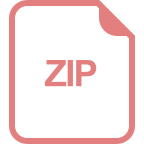