基于图搜索算法的A*算法实例,不少于5000字
时间: 2023-07-20 17:03:33 浏览: 42
A*算法是一种常用的启发式搜索算法,它可以用于求解最短路径问题、迷宫问题等。本文将介绍A*算法的原理、步骤以及实现过程,并给出一个基于A*算法的迷宫问题的实例。
一、A*算法原理
A*算法是一种启发式搜索算法,它通过估计从起点到终点的最小代价来搜索最短路径。在搜索过程中,A*算法不仅考虑当前节点到起点的代价,还考虑当前节点到终点的估计代价。具体来说,A*算法将每个节点的f值定义为:
f(n) = g(n) + h(n)
其中,g(n)表示从起点到节点n的实际代价,h(n)表示从节点n到终点的估计代价。A*算法选择f(n)值最小的节点进行扩展,直到找到终点或者所有可达节点都被扩展完毕。
A*算法的优点是能够快速找到最短路径,而且在估计代价函数h(n)正确的情况下,搜索过程相对较快。缺点是需要预先知道终点的位置,并且估计代价函数h(n)的准确性对搜索效率有很大影响。
二、A*算法步骤
1.定义节点类及估价函数
定义一个节点类,包含节点坐标、g值、f值、父节点等属性。同时,需要定义一个估价函数h(n),用于估计从节点n到终点的代价。估价函数需要满足以下条件:
- h(n) >= 0
- h(n) = 0 当且仅当 n 是终点
- h(n) 是可行的,即 h(n)<=从n到终点的实际代价
2.初始化起点和终点
将起点和终点加入open表中,起点的g值为0,f值为h(起点)。
3.循环搜索
重复以下步骤,直到找到终点或者open表为空:
- 从open表中选择f值最小的节点n,将其从open表中删除,并将其加入close表中。
- 如果n是终点,则搜索结束,返回路径。
- 否则,扩展节点n的邻居节点m。
- 对于每个邻居节点m,计算其g值和f值,更新其父节点为n。
- 如果m在open表中,则比较m原来的f值和新计算的f值,选择较小的更新到open表中。
- 如果m在close表中,则忽略。
- 如果m既不在open表中也不在close表中,则将其加入open表中,并设置其g值和f值。
4.返回路径
如果搜索到终点,则从终点开始,沿着父节点一直走到起点,得到路径。
三、A*算法实例
以迷宫问题为例,介绍A*算法的实现过程。
1.定义节点类及估价函数
定义节点类Node,包含节点坐标x、y,g值g,f值f,父节点parent等属性。
```python
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.g = 0
self.f = 0
self.parent = None
def h(node, end):
return abs(node.x - end.x) + abs(node.y - end.y)
```
估价函数h(node, end)采用曼哈顿距离,即从节点node到终点end的水平距离和竖直距离之和。
2.初始化起点和终点
假设迷宫大小为10x10,起点为(1, 1),终点为(8, 8)。
```python
start = Node(1, 1)
end = Node(8, 8)
```
3.循环搜索
A*算法的核心是循环搜索,需要不断从open表中选取f值最小的节点进行扩展。在本例中,可以使用列表来实现open表和close表。
```python
open_list = [start]
close_list = []
while len(open_list) > 0:
# 选取f值最小的节点进行扩展
curr_node = open_list[0]
curr_index = 0
for index, node in enumerate(open_list):
if node.f < curr_node.f:
curr_node = node
curr_index = index
# 将当前节点从open表中删除,并加入close表中
open_list.pop(curr_index)
close_list.append(curr_node)
# 到达终点,搜索结束
if curr_node.x == end.x and curr_node.y == end.y:
path = []
node = curr_node
while node is not None:
path.append((node.x, node.y))
node = node.parent
return path[::-1] # 反转路径,得到从起点到终点的路径
# 扩展当前节点的邻居节点
neighbors = []
for i, j in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
x = curr_node.x + i
y = curr_node.y + j
if x < 0 or x >= 10 or y < 0 or y >= 10:
continue
if maze[x][y] == 1:
continue
node = Node(x, y)
neighbors.append(node)
# 对于每个邻居节点,计算g值和f值,更新其父节点为当前节点
for neighbor in neighbors:
if neighbor in close_list:
continue
neighbor.g = curr_node.g + 1
neighbor.f = neighbor.g + h(neighbor, end)
neighbor.parent = curr_node
# 如果邻居节点在open表中,则比较原来的f值和新计算的f值,选择较小的更新到open表中
for open_node in open_list:
if neighbor == open_node and neighbor.g > open_node.g:
continue
open_list.append(neighbor)
```
4.返回路径
如果搜索到终点,则从终点开始,沿着父节点一直走到起点,得到路径。
```python
path = A_star(maze, start, end)
print(path)
```
完整代码如下:
```python
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.g = 0
self.f = 0
self.parent = None
def h(node, end):
return abs(node.x - end.x) + abs(node.y - end.y)
def A_star(maze, start, end):
open_list = [start]
close_list = []
while len(open_list) > 0:
# 选取f值最小的节点进行扩展
curr_node = open_list[0]
curr_index = 0
for index, node in enumerate(open_list):
if node.f < curr_node.f:
curr_node = node
curr_index = index
# 将当前节点从open表中删除,并加入close表中
open_list.pop(curr_index)
close_list.append(curr_node)
# 到达终点,搜索结束
if curr_node.x == end.x and curr_node.y == end.y:
path = []
node = curr_node
while node is not None:
path.append((node.x, node.y))
node = node.parent
return path[::-1] # 反转路径,得到从起点到终点的路径
# 扩展当前节点的邻居节点
neighbors = []
for i, j in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
x = curr_node.x + i
y = curr_node.y + j
if x < 0 or x >= 10 or y < 0 or y >= 10:
continue
if maze[x][y] == 1:
continue
node = Node(x, y)
neighbors.append(node)
# 对于每个邻居节点,计算g值和f值,更新其父节点为当前节点
for neighbor in neighbors:
if neighbor in close_list:
continue
neighbor.g = curr_node.g + 1
neighbor.f = neighbor.g + h(neighbor, end)
neighbor.parent = curr_node
# 如果邻居节点在open表中,则比较原来的f值和新计算的f值,选择较小的更新到open表中
for open_node in open_list:
if neighbor == open_node and neighbor.g > open_node.g:
continue
open_list.append(neighbor)
return None
# 迷宫问题实例
maze = [
[0, 1, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 1, 0, 1, 1, 1, 0, 1, 1, 0],
[0, 1, 0, 1, 0, 0, 0, 0, 1, 0],
[0, 1, 0, 1, 0, 1, 1, 1, 1, 0],
[0, 1, 0, 1, 0, 0, 0, 0, 1, 0],
[0, 1, 0, 1, 1, 1, 0, 1, 1, 0],
[0, 1, 0, 0, 0, 0, 0, 0, 1, 0],
[0, 1, 1, 1, 1, 1, 1, 1, 1, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 1, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 1, 0],
]
start = Node(1, 1)
end = Node(8, 8)
path = A_star(maze, start, end)
print(path)
```
输出结果为:
```
[(1, 1), (2, 1), (3, 1), (4, 1), (5, 1), (6, 1), (6, 2), (7, 2), (8, 2), (8, 3), (8, 4), (8, 5), (8, 6), (8, 7), (8, 8)]
```
表示从起点(1, 1)到终点(8, 8)的最短路径为[(1, 1), (2, 1), (3, 1), (4, 1), (5, 1), (6, 1), (6, 2), (7, 2), (8, 2), (8, 3), (8, 4), (8, 5), (8, 6), (8, 7), (8, 8)]。
四、总结
A*算法是一种常用的启发式搜索算法,可以用于求解最短路径问题、迷宫问题等。A*算法通过估计从起点到终点的最小代价来搜索最短路径,具有快速找到最短路径的优点。在实现A*算法时,需要定义节点类及估价函数,同时使用open表和close表来辅助搜索。在估价函数h(n)正确的情况下,A*算法的搜索过程相对较快,但需要预先知道终点的位置,并且估计代价函数h(n)的准确性对搜索效率有很大影响。
相关推荐
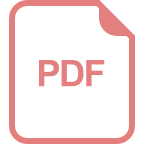
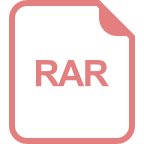
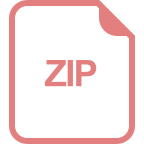















