c++通过链接下载抖音视频
时间: 2023-08-04 09:03:50 浏览: 208
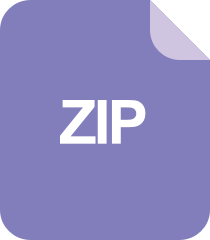
vc++ 链接下载的实现
我作为 AI 并不能执行下载操作,但是可以给你一些思路。首先,要使用 C++ 下载抖音视频需要了解抖音视频的下载链接地址。抖音视频下载链接一般是通过抓包获取的。然后,可以使用 C++ 的网络编程相关函数如 `socket`、`connect`、`send`、`recv` 等来进行网络通信,发送 HTTP 请求并接收响应,从响应中解析出视频的下载链接地址,最后使用 C++ 文件操作函数如 `fopen`、`fwrite`、`fclose` 等将视频文件保存到本地。需要注意的是,下载视频涉及到版权问题,需要遵守相关法律法规。
阅读全文
相关推荐














