c++通过抖音分享链接获取视频链接
时间: 2023-08-03 12:04:26 浏览: 222
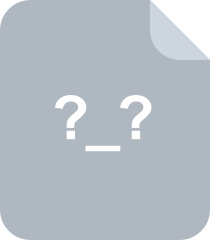
基于C++的获取视频前景
在 C++ 中,你可以使用网络请求库(如 cURL 或 libcurl)来发送 HTTP 请求,然后解析响应以获取抖音分享链接对应的视频链接。以下是一个基本的示例代码:
```c++
#include <curl/curl.h>
#include <iostream>
#include <regex>
// 回调函数,用于接收 HTTP 响应
static size_t write_callback(char* ptr, size_t size, size_t nmemb, std::string* data) {
data->append(ptr, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string url = "https://www.douyin.com/video/xxxxxx";
std::string response;
curl = curl_easy_init();
if (curl) {
// 设置请求的 URL
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
// 设置回调函数,接收 HTTP 响应
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
// 设置接收响应数据的缓冲区
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
// 发送 HTTP 请求
res = curl_easy_perform(curl);
// 关闭 Curl
curl_easy_cleanup(curl);
if (res == CURLE_OK) {
// 使用正则表达式提取视频链接
std::regex pattern("\"playAddr\":\"(.*?)\"");
std::smatch match;
if (std::regex_search(response, match, pattern)) {
std::cout << "Video URL: " << match[1].str() << std::endl;
} else {
std::cout << "Failed to extract video URL." << std::endl;
}
} else {
std::cout << "HTTP request failed: " << curl_easy_strerror(res) << std::endl;
}
}
return 0;
}
```
需要注意的是,抖音的视频链接可能会有防盗链限制,需要在请求头中添加相应的 Referer 和 User-Agent。你需要在 `curl_easy_setopt` 中添加以下两行代码:
```c++
// 设置 Referer
curl_easy_setopt(curl, CURLOPT_REFERER, "https://www.douyin.com/");
// 设置 User-Agent
curl_easy_setopt(curl, CURLOPT_USERAGENT, "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3");
```
其中 `Referer` 为抖音的域名,`User-Agent` 可以使用浏览器的 User-Agent。
阅读全文
相关推荐







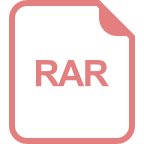
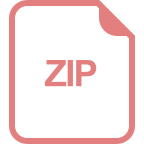
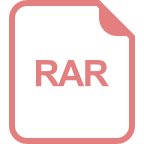
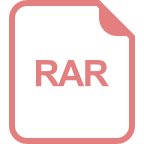
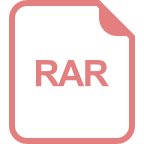
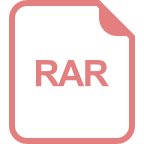