PyTorch 1.9.0的指令
时间: 2024-05-11 11:18:10 浏览: 123
以下是PyTorch 1.9.0的一些常用指令:
1. 创建张量:
```python
import torch
# 创建一个大小为2x3的浮点型张量
x = torch.FloatTensor([[1, 2, 3], [4, 5, 6]])
print(x)
```
2. 张量属性:
```python
# 张量的形状
print(x.shape)
# 张量的数据类型
print(x.dtype)
# 张量的设备类型(CPU或GPU)
print(x.device)
```
3. 张量操作:
```python
# 张量相加
y = torch.FloatTensor([[7, 8, 9], [10, 11, 12]])
z = x + y
print(z)
# 张量点乘
a = torch.FloatTensor([1, 2, 3])
b = torch.FloatTensor([4, 5, 6])
c = torch.dot(a, b)
print(c)
# 张量矩阵乘法
m1 = torch.FloatTensor([[1, 2], [3, 4]])
m2 = torch.FloatTensor([[5, 6], [7, 8]])
m3 = torch.mm(m1, m2)
print(m3)
```
4. 张量梯度求解:
```python
# 创建一个需要求梯度的张量
x = torch.randn(3, requires_grad=True)
# 定义一个计算图
y = x * 2 + 1
z = y.mean()
# 计算张量y的梯度
y.backward()
# 打印张量x的梯度
print(x.grad)
```
5. 使用GPU:
```python
# 将张量转移到GPU设备上
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
x = x.to(device)
# 在GPU上进行张量操作
y = x * 2 + 1
# 将结果转移到CPU设备上
y = y.to("cpu")
```
阅读全文
相关推荐
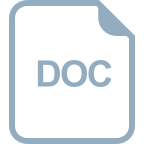
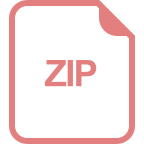




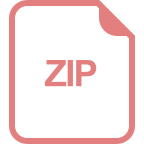
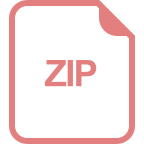
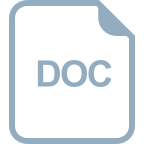
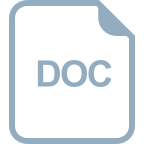
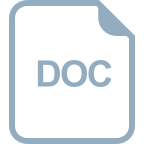



