# 获取当前工作目录 path = os.getcwd() # 连接两个文件路径 filename = os.path.join(path, 'info.txt')。这是什么意思
时间: 2024-06-06 22:06:06 浏览: 10
这段代码是使用Python内置的OS模块对文件进行操作的示例。首先,使用`os.getcwd()`方法获取当前工作目录的路径,并将其存储在变量`path`中。然后,使用`os.path.join()`方法将当前工作目录的路径与文件名`info.txt`连接起来,形成一个完整的文件路径,并将其存储在变量`filename`中。这个过程可以用来创建、打开、读写文件等操作。
相关问题
path = os.path.join(os.getcwd(), "temp", image._blob.filename) AttributeError: 'InlineShape' object has no attribute '_blob'
这个错误是因为在`InlineShape`对象中没有`_blob`属性。可能是因为你尝试使用一个带有图片的`InlineShape`对象,但是该对象中并没有`_blob`属性。
你可以尝试以下几个步骤来解决这个问题:
1. 检查你是否正确地获取了`InlineShape`对象。尝试打印该对象并检查它是否是你想要的对象。
2. 确认该对象是否真的包含了图片。如果不是,那么可能就没有`_blob`属性。
3. 如果你确定该对象应该包含图片,那么可能是你的代码有问题。尝试检查你的代码并确定是否有任何错误。
如果你还是无法解决问题,可以提供更多代码和上下文,以便更好地理解问题。
inStr = "" with open(os.path.abspath(os.path.join(os.getcwd(),"sv", filename )) ,"r") as fn: inStr = fn.read()
你的代码是用来读取一个文件的内容并将其存储在变量 `inStr` 中。这段代码使用了 Python 的 `open()` 函数来打开文件,`os.path.abspath()` 函数来获取文件的绝对路径,`os.path.join()` 函数来拼接文件路径,以及 `os.getcwd()` 函数来获取当前工作目录。
其中,`os.path.abspath(os.path.join(os.getcwd(),"sv", filename ))` 的作用是获取文件的绝对路径,其中 `filename` 是文件名。
然后,代码使用 `with open(...) as fn:` 的语法来打开文件,并将文件对象保存在变量 `fn` 中。接着使用 `fn.read()` 方法来读取文件的全部内容,并将其赋值给变量 `inStr`。
这样,变量 `inStr` 就存储了文件的内容。请注意,这段代码假设文件位于一个名为 "sv" 的子目录中。
相关推荐
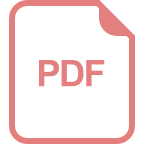
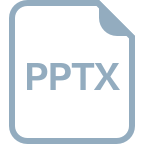
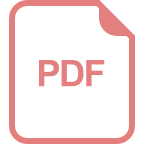













