定义一个形状类(Shape)方法:计算周长,计算面积 子类: 矩形类(Rectangle) :额外的方法:differ() 计算长宽差 圆形类(Circle) 三角形类(Triangle) 正方形类(Square) 矩形的子类 生成几个不同的形状对象,放在一个 Shape 类型的数组里,分别求每个形状的周长和面积。 如果形状对象是一个矩形,且不是正方形,则计算长宽差。java语言
时间: 2024-01-07 09:05:17 浏览: 112
好的,以下是相应的Java代码示例:
```
public class Shape {
public double calculatePerimeter() {
return 0.0;
}
public double calculateArea() {
return 0.0;
}
}
public class Rectangle extends Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double calculatePerimeter() {
return 2 * (length + width);
}
public double calculateArea() {
return length * width;
}
public double differ() {
return Math.abs(length - width);
}
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
public double calculateArea() {
return Math.PI * radius * radius;
}
}
public class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
public double calculatePerimeter() {
return 3 * base;
}
public double calculateArea() {
return 0.5 * base * height;
}
}
public class Square extends Rectangle {
public Square(double side) {
super(side, side);
}
}
public class Main {
public static void main(String[] args) {
Shape[] shapes = {new Rectangle(4, 5), new Circle(3), new Triangle(6, 8), new Square(5)};
for (Shape shape : shapes) {
System.out.println("Perimeter: " + shape.calculatePerimeter());
System.out.println("Area: " + shape.calculateArea());
if (shape instanceof Rectangle && ((Rectangle) shape).differ() != 0) {
System.out.println("Difference: " + ((Rectangle) shape).differ());
}
System.out.println();
}
}
}
```
这个代码会输出每个形状的周长和面积,如果是矩形且不是正方形,还会输出长宽差。你可以根据需要进行修改和扩展。
阅读全文
相关推荐




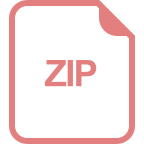
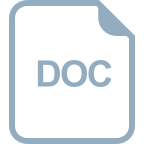
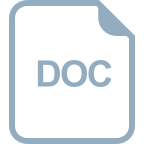
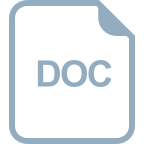







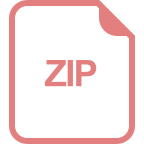