羊狼猎人过河flash
时间: 2023-08-21 16:00:38 浏览: 205
《羊狼猎人过河flash》是一款益智游戏。游戏中,你需要控制一个猎人将一只羊和一只狼过河,但是船的载重量有限,你必须注意安全性和策略性,避免羊和狼之间发生冲突。
首先,你需要将猎人载满船,跨过河岸并将猎人放下。然后,你可以将狼载上船,将猎人带回起点,然后将狼放下。接下来,你需要将羊载上船并跨过河岸,但是要确保狼不会吃掉羊。最后,将羊带回起点,完成整个过河任务。
在游戏中,你需要考虑到狼和羊之间的互动关系。如果你把狼和羊留在一起,狼会吃掉羊。因此,在每个行动之前都要仔细分析和规划,确保羊和狼安全地过河而没有冲突发生。
这款游戏不仅需要逻辑思维和解决问题的能力,还需要一定的动态协调能力。你需要判断船的载重量是否合适,选择正确的顺序将物品带过河,并灵活地应对情况的变化。
《羊狼猎人过河flash》是一款有趣的游戏,不仅可以帮助你锻炼逻辑和推理能力,还能培养你的策略性思维和应变能力。通过与羊、狼和猎人之间的互动,你可以更好地理解问题的本质,并学会制定合适的解决方案。
相关问题
猎人过河分支限界法C语言
以下是使用C语言实现猎人过河问题的分支限界法的伪代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX 100 // 状态空间树的最大节点数
typedef struct node {
int side[2][3]; // side[0]表示左岸,side[1]表示右岸,0表示猎人,1表示狗,2表示兔子
int boat; // 船的位置,0表示左岸,1表示右岸
int depth; // 节点的深度
int f; // 优先级,即启发函数的值
} Node;
typedef struct queue {
Node data[MAX];
int front, rear;
} Queue;
void init(Queue *q) { // 初始化队列
q->front = q->rear = 0;
}
int is_empty(Queue *q) { // 判断队列是否为空
return q->front == q->rear;
}
int is_full(Queue *q) { // 判断队列是否已满
return (q->rear + 1) % MAX == q->front;
}
void enqueue(Queue *q, Node n) { // 入队
if (is_full(q)) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = n;
q->rear = (q->rear + 1) % MAX;
}
Node dequeue(Queue *q) { // 出队
Node n;
if (is_empty(q)) {
printf("Queue is empty.\n");
return n;
}
n = q->data[q->front];
q->front = (q->front + 1) % MAX;
return n;
}
int is_goal(Node n) { // 判断是否到达目标状态
return n.side[0][0] == 0 && n.side[0][1] == 0 && n.side[0][2] == 0;
}
int is_valid(Node n) { // 判断状态是否合法
if (n.side[0][1] == n.side[0][2] && n.side[0][1] != n.side[0][0]) return 0; // 狗吃兔子
if (n.side[1][1] == n.side[1][2] && n.side[1][1] != n.side[1][0]) return 0; // 狗离开猎人回家
return 1;
}
int heuristic(Node n) { // 启发函数,估计当前状态到达目标状态的代价
int cost = 0;
for (int i = 0; i < 3; i++) {
if (n.side[0][i] == 1) cost++; // 左岸狗和兔子的数量
if (n.side[1][i] == 1) cost++; // 右岸狗和兔子的数量
}
return cost;
}
void print(Node n) { // 打印状态
printf("%c %c %c %c\n", n.side[0][0] ? 'H' : '_', n.side[0][1] ? 'D' : '_', n.side[0][2] ? 'R' : '_', n.boat ? '>' : '<');
printf("%c %c %c\n", n.side[1][0] ? 'H' : '_', n.side[1][1] ? 'D' : '_', n.side[1][2] ? 'R' : '_');
printf("Depth: %d\n", n.depth);
printf("f: %d\n", n.f);
}
void expand(Node n, Queue *q) { // 扩展当前状态,生成子节点
Node child;
for (int i = 0; i < 3; i++) {
if (n.side[n.boat][i] == 0) continue; // 物品不在船上
child = n;
child.side[n.boat][i] = 0; // 物品从船上下来
child.side[1 - n.boat][i] = 1; // 物品到达对岸
child.boat = 1 - n.boat; // 船到达对岸
child.depth = n.depth + 1; // 子节点深度加一
if (is_valid(child)) { // 如果子状态合法
child.f = child.depth + heuristic(child); // 计算优先级
enqueue(q, child); // 入队
}
}
}
void search(Node initial) { // 分支限界法搜索
Queue q;
Node n, child;
init(&q);
enqueue(&q, initial);
while (!is_empty(&q)) {
n = dequeue(&q);
if (is_goal(n)) {
printf("Goal reached:\n");
print(n);
return;
}
expand(n, &q);
}
printf("Goal not found.\n");
}
int main() {
Node initial = { { { 1, 1, 1 }, { 0, 0, 0 } }, 0, 0, heuristic(initial) };
search(initial);
return 0;
}
```
以上是使用C语言实现猎人过河问题的分支限界法的伪代码,其中包含队列的实现、状态的表示、启发函数的计算、状态扩展和搜索等部分。可以根据实际情况进行调整和修改。
用c++写一下猎人过河问题
好的,以下是用C++实现猎人过河问题的代码:
```c++
#include <iostream>
using namespace std;
int main() {
bool hunter = true; // 猎人所在岸
bool dog = true; // 狗所在岸
bool chicken = true; // 鸡所在岸
bool corn = true; // 玉米所在岸
while (true) {
// 输出当前状态
cout << "Hunter: " << (hunter ? "Left" : "Right") << endl;
cout << "Dog: " << (dog ? "Left" : "Right") << endl;
cout << "Chicken: " << (chicken ? "Left" : "Right") << endl;
cout << "Corn: " << (corn ? "Left" : "Right") << endl;
// 检查是否已经成功
if (!hunter && !dog && !chicken && !corn) {
cout << "All transported successfully!" << endl;
break;
}
// 猎人运送
bool canTransport = false; // 是否可以运送
if (hunter == dog) { // 猎人和狗在同一岸
if (hunter == chicken) { // 猎人和鸡在同一岸
canTransport = true; // 可以运送玉米
} else { // 猎人和鸡不在同一岸
canTransport = true; // 可以运送鸡
}
} else { // 猎人和狗不在同一岸
if (hunter == chicken) { // 猎人和鸡在同一岸
canTransport = true; // 可以运送狗
}
}
if (canTransport) { // 可以运送
int choice;
cout << "What to transport (1:hunter, 2:dog, 3:chicken, 4:corn)? ";
cin >> choice; // 输入运送的物品编号
switch (choice) {
case 1:
hunter = !hunter;
break;
case 2:
dog = !dog;
break;
case 3:
chicken = !chicken;
break;
case 4:
corn = !corn;
break;
default:
cout << "Invalid choice!" << endl;
break;
}
} else { // 不可以运送
cout << "Cannot transport!" << endl;
}
}
return 0;
}
```
运行这个程序,你可以与计算机互动地解决猎人过河问题。
阅读全文
相关推荐
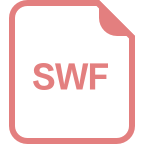











